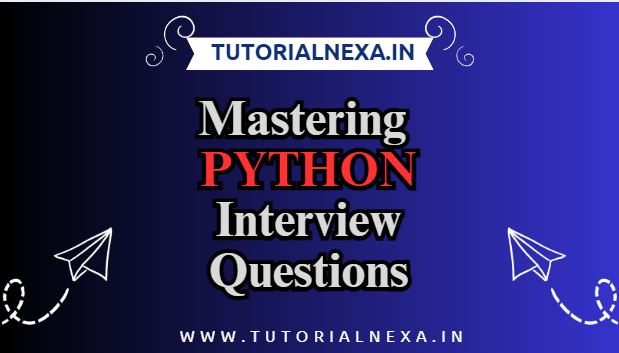
1. What is the output of the following Python code?
Python code :
x = 5 y = 2 result = x // y print(result)
Options:
- 2.5
- 2.0
- 2
- 3
Correct Answer: 3. 2
Explanation: The //
operator performs floor division, which returns the largest integer less than or equal to the division of the operands. In this case, 5 divided by 2 is 2 with a remainder, so the output is 2.*
2. Which of the following statements is true regarding Python’s list?
Options:
- Lists are immutable.
- Lists can contain elements of different data types.
- Lists can only be accessed using numeric indices.
- Lists can have a fixed size.
Correct Answer: 2. Lists can contain elements of different data types.
Explanation: Python lists can hold elements of different data types, making them versatile. Unlike some other languages, Python lists are mutable, meaning you can modify them after creation.*
3. What will be the output of the following code?
python code:
numbers = [1, 2, 3, 4, 5] squared = [num**2 for num in numbers if num % 2 == 0] print(squared)
Options:
- [1, 4, 9, 16, 25]
- [4, 16]
- [1, 9, 25]
- [1, 4, 16]
Correct Answer: 2. [4, 16]
Explanation: The list comprehension generates a new list containing the squares of even numbers from the original list. Thus, the output is [4, 16]
.*
4. What does the pass
statement do in Python?
Options:
- Exits the program.
- Skips the current iteration in a loop.
- Declares a variable without assigning a value.
- Raises an exception.
Correct Answer: 2. Skips the current iteration in a loop.
Explanation: The pass
statement in Python is a no-operation statement. It is used when a statement is syntactically required, but you don’t want to execute any code. It is often used as a placeholder and does nothing when executed.*
5. How can you open a file named “example.txt” in Python for reading and writing?
Options:
file = open("example.txt", "r")
file = open("example.txt", "w")
file = open("example.txt", "rw")
file = open("example.txt", "a")
Correct Answer: 4. file = open("example.txt", "a")
Explanation: To open a file in Python for both reading and writing, you can use the “a” mode (append). This allows you to read and write to the file without truncating it.*
6. What is the purpose of the __init__
method in a Python class?
Options:
- It is used to initialize the class object.
- It is a reserved method for private initialization.
- It is used to destroy the class object.
- It is a special method for defining class attributes.
Correct Answer:
- It is used to initialize the class object.
Explanation: The __init__
method is a special method in Python classes that is automatically called when an object is created. It is used to initialize the attributes of the class.*
7. In Python, what is the purpose of the else
clause in a try-except block?
Options:
- It is executed if an exception occurs.
- It is executed if no exception occurs.
- It is used to handle specific exceptions.
- It is used to raise a custom exception.
Correct Answer: 2. It is executed if no exception occurs.
Explanation: The else
clause in a try-except block is executed if no exceptions are raised in the corresponding try block.*
8. What is the purpose of the super()
function in Python?
Options:
- It calls the parent class’s constructor.
- It returns the current instance of the class.
- It is used to create a new instance of a class.
- It is a keyword for inheritance.
Correct Answer:
- It calls the parent class’s constructor.
Explanation: The super()
function is used to call a method from the parent class. It is commonly used in the __init__
method of a subclass to invoke the constructor of the parent class.*
9. What is the purpose of the finally
clause in a try-except block in Python?
Options:
- It is executed if an exception occurs.
- It is executed if no exception occurs.
- It is always executed, regardless of whether an exception occurs or not.
- It is used to handle specific exceptions.
Correct Answer: 3. It is always executed, regardless of whether an exception occurs or not.
Explanation: The finally
clause in a try-except block is used to define cleanup actions that must be executed, regardless of whether an exception is raised or not.*
10. How can you concatenate two lists in Python?
Options:
list1.join(list2)
list1 + list2
concat(list1, list2)
merge(list1, list2)
Correct Answer: 2. list1 + list2
Explanation: The +
operator is used for list concatenation in Python. It combines the elements of two lists to create a new list.*
11. What is the purpose of the break
statement in a loop in Python?
Options:
- It ends the entire program.
- It skips the current iteration and continues with the next one.
- It terminates the loop and transfers control to the next statement after the loop.
- It raises an exception.
Correct Answer: 3. It terminates the loop and transfers control to the next statement after the loop.
Explanation: The break
statement is used to exit a loop prematurely. It terminates the loop and transfers control to the next statement after the loop.*
12. What does the __str__
method do in Python?
Options:
- It converts an object to a string representation.
- It creates a new string object.
- It is used for string formatting.
- It is a reserved method for system strings.
Correct Answer:
- It converts an object to a string representation.
Explanation: The __str__
method is a special method in Python that is called when the str()
function is used on an object. It should return a string representation of the object.*
13. In Python, what is the purpose of the enumerate
function?
Options:
- It counts the number of elements in a list.
- It returns the index and value of each element in an iterable.
- It filters elements based on a given condition.
- It reverses the order of elements in a list.
Correct Answer: 2. It returns the index and value of each element in an iterable.
Explanation: The enumerate
function in Python is used to iterate over a sequence (list, tuple, etc.) along with its index, providing both the index and the value of each element.*
14. What is the purpose of the lambda
function in Python?
Options:
- It is used for declaring global variables.
- It is a reserved keyword for anonymous functions.
- It defines a new class in Python.
- It is used to handle exceptions.
Correct Answer: 2. It is a reserved keyword for anonymous functions.
Explanation: A lambda
function is a concise way to create anonymous functions in Python. It is often used for short, one-time operations where a full function definition is unnecessary.*
15. How can you check if a key is present in a dictionary in Python?
Options:
key in dict
dict.contains(key)
dict[key] != None
key.exists(dict)
Correct Answer:
key in dict
Explanation: The in
keyword is used to check if a key is present in a dictionary in Python. It returns True
if the key is found, and False
otherwise.*
16. What is the purpose of the zip
function in Python?
Options:
- It compresses files into a zip archive.
- It creates an iterator that aggregates elements from multiple iterables.
- It extracts files from a zip archive.
- It calculates the absolute value of a number.
Correct Answer: 2. It creates an iterator that aggregates elements from multiple iterables.
Explanation: The zip
function in Python is used to combine elements from multiple iterables (e.g., lists, tuples) into tuples. It creates an iterator that generates tuples containing elements from the input iterables, allowing for parallel iteration.*
Explanation: The zip
function in Python is used to combine elements from multiple iterables (e.g., lists, tuples) into tuples. It creates an iterator that generates tuples containing elements from the input iterables, allowing for parallel iteration.*
26. How can you check if a variable is of a certain data type in Python?
Options:
variable.is_type()
type(variable) == "desired_type"
isinstance(variable, desired_type)
variable.check_type(desired_type)
Correct Answer: 3. isinstance(variable, desired_type)
Explanation: The isinstance()
function in Python is used to check if a variable is of a certain data type. It returns True
if the variable is an instance of the specified type, otherwise False
.*
27. What is the purpose of the __iter__
method in Python?
Options:
- It initializes an iterator object.
- It is used to iterate over elements in a list.
- It returns an iterator for the object.
- It is a reserved method for system iteration.
Correct Answer: 3. It returns an iterator for the object.
Explanation: The __iter__
method is used to define how an object should create an iterator. It returns an iterator object, which can be used to iterate over the elements of the object.*
28. How do you remove the last element from a list in Python?
Options:
list.pop()
list.remove(-1)
list.delete(-1)
list[-1] = None
Correct Answer:
list.pop()
Explanation: The pop()
method in Python, without specifying an index, removes and returns the last element from a list.*
29. What is the purpose of the ord()
function in Python?
Options:
- It calculates the absolute value of a number.
- It converts a character to its Unicode code point.
- It rounds a floating-point number to the nearest integer.
- It is used for bitwise operations.
Correct Answer: 2. It converts a character to its Unicode code point.
Explanation: The ord()
function in Python returns the Unicode code point of a given character. It is often used in conjunction with the chr()
function.*
30. How can you check if a file exists in Python before attempting to open it?
Options:
if file.exists()
if os.file_exists()
if os.path.exists(file)
if file.check()
Correct Answer: 3. if os.path.exists(file)
Explanation: The os.path.exists()
function in Python is used to check if a file or directory exists at the specified path. It returns True
if the path exists, otherwise False
.*
31. What is the purpose of the random.choice()
function in Python?
Options:
- It generates a random integer.
- It selects a random element from a list.
- It shuffles the elements of a list.
- It generates a random floating-point number.
Correct Answer: 2. It selects a random element from a list.
Explanation: The random.choice()
function in Python is used to select a random element from a sequence, such as a list.*
32. How can you convert a list to a tuple in Python?
Options:
tuple(list)
list.to_tuple()
convert_to_tuple(list)
list(tuple)
Correct Answer:
tuple(list)
Explanation: The tuple()
constructor in Python can be used to convert a list to a tuple.*
33. What does the __str__
method do in a Python class?
Options:
- It converts an object to a string representation.
- It creates a new string object.
- It is used for string formatting.
- It is a reserved method for system strings.
Correct Answer:
- It converts an object to a string representation.
Explanation: The __str__
method in a Python class is called when the str()
function is used on an object. It should return a string representation of the object.*
34. How can you reverse the order of elements in a list in Python?
Options:
list.reverse()
reversed(list)
list.sort(reverse=True)
list.flip()
Correct Answer: 2. reversed(list)
Explanation: The reversed()
function in Python returns a reverse iterator, which can be used to iterate over the elements of a sequence in reverse order. To convert it back to a list, you can use list(reversed(my_list))
or use slicing, my_list[::-1]
.*
35. What is the purpose of the break
statement in a loop in Python?
Options:
- It ends the entire program.
- It skips the current iteration and continues with the next one.
- It terminates the loop and transfers control to the next statement after the loop.
- It raises an exception.
Correct Answer: 3. It terminates the loop and transfers control to the next statement after the loop.
Explanation: The break
statement in Python is used to exit a loop prematurely. It terminates the loop and transfers control to the next statement after the loop.*
36. What is the purpose of the __len__
method in Python?
Options:
- It returns the length of a list or tuple.
- It is a reserved method for system length calculations.
- It is used to define the length of a class.
- It is a special method to access the length of an object.
Correct Answer:
- It returns the length of a list or tuple.
Explanation: The __len__
method in Python is a special method that is called when the built-in len()
function is used on an object. It should return the length of the object.*
37. How can you concatenate two dictionaries in Python?
Options:
dict1.append(dict2)
dict1 + dict2
dict1.extend(dict2)
dict1.update(dict2)
Correct Answer: 4. dict1.update(dict2)
Explanation: To concatenate two dictionaries in Python, you can use the update()
method, which adds the key-value pairs from one dictionary to another.*
38. What is the purpose of the try
and except
blocks in Python?
Options:
- They define a new function.
- They handle errors and exceptions.
- They create a loop.
- They are reserved keywords for system-level operations.
Correct Answer: 2. They handle errors and exceptions.
Explanation: The try
and except
blocks in Python are used for exception handling. Code that may raise an exception is placed inside the try
block, and the handling of the exception is done in the except
block.*
39. How can you convert a string to an integer in Python?
Options:
int(string)
string.to_int()
convert_to_int(string)
integer(string)
Correct Answer:
int(string)
Explanation: The int()
function in Python is used to convert a string to an integer.*
40. What is the purpose of the pass
statement in Python?
Options:
- It is used to end the program.
- It skips the current iteration in a loop.
- It is a placeholder for future code.
- It raises an exception.
Correct Answer: 3. It is a placeholder for future code.
Explanation: The pass
statement in Python is often used as a placeholder where syntactically some code is required but no action is desired.*
41. How can you round a floating-point number to a specified number of decimal places in Python?
Options:
round(number, decimals)
number.round(decimals)
round(number, places)
number.toFixed(decimals)
Correct Answer:
round(number, decimals)
Explanation: The round()
function in Python is used to round a floating-point number to the specified number of decimal places.*
42. What is the purpose of the with
statement in Python?
Options:
- It defines a new context manager.
- It creates a loop.
- It handles exceptions.
- It is a reserved keyword for file operations.
Correct Answer:
- It defines a new context manager.
Explanation: The with
statement in Python is used to create a context manager, which simplifies resource management, such as file handling, by automatically taking care of setup and teardown operations.*
43. What is the purpose of the filter()
function in Python?
Options:
- It filters elements based on a given condition.
- It creates a new list containing the unique elements of an existing list.
- It applies a function to all the elements of an iterable.
- It is used for filtering files in a directory.
Correct Answer:
- It filters elements based on a given condition.
Explanation: The filter()
function in Python is used to filter elements from an iterable based on a specified function or condition.*
44. How can you convert a tuple to a list in Python?
Options:
list.convert(tuple)
tuple.to_list()
list(tuple)
convert_to_list(tuple)
Correct Answer: 3. list(tuple)
Explanation: The list()
constructor in Python can be used to convert a tuple to a list.*
45. What is the purpose of the __eq__
method in Python?
Options:
- It is a reserved method for equality comparisons.
- It defines a new class attribute.
- It is used for exception handling.
- It creates a new instance of a class.
Correct Answer:
- It is a reserved method for equality comparisons.
Explanation: The __eq__
method in Python is a special method used for defining the behavior of the equality operator (==
) for instances of a class.*
46. How can you check if a number is an integer in Python?
Options:
number.is_integer()
integer(number)
isinteger(number)
type(number) == int
Correct Answer:
number.is_integer()
Explanation: The is_integer()
method in Python can be used to check if a floating-point number represents an integer.*
47. What is the purpose of the del
statement in Python?
Options:
- It is used to delete a file.
- It removes an element from a list.
- It deletes a variable or object.
- It is a reserved keyword for declaring classes.
Correct Answer: 3. It deletes a variable or object.
Explanation: The del
statement in Python is used to delete a variable or object. It can also be used to delete elements from a list or a dictionary.*
48. How can you convert a list of strings to a single string in Python?
Options:
str(list)
"".join(list)
list.convert_to_string()
string(list)
Correct Answer: 2. "".join(list)
Explanation: The "".join(list)
expression in Python can be used to concatenate the elements of a list of strings into a single string.*
49. What is the purpose of the zip
function in Python?
Options:
- It compresses files into a zip archive.
- It creates an iterator that aggregates elements from multiple iterables.
- It extracts files from a zip archive.
- It calculates the absolute value of a number.
Correct Answer: 2. It creates an iterator that aggregates elements from multiple iterables.
Explanation: The zip
function in Python is used to combine elements from multiple iterables (e.g., lists, tuples) into tuples. It creates an iterator that generates tuples containing elements from the input iterables, allowing for parallel iteration.*
50. What is the purpose of the os
module in Python?
Options:
- It is used for mathematical calculations.
- It provides a way to interact with the operating system.
- It defines basic data types in Python.
- It is a reserved module for string operations.
Correct Answer: 2. It provides a way to interact with the operating system.
Explanation: The os
module in Python provides a way to interact with the operating system, allowing you to perform various system-related tasks, such as file and directory operations.*
Explanation: The os
module in Python provides a way to interact with the operating system, allowing you to perform various system-related tasks, such as file and directory operations.*
61. What is the purpose of the chr
function in Python?
Options:
- It calculates the absolute value of a number.
- It converts a character to its Unicode code point.
- It generates a character from a Unicode code point.
- It is used for character mapping.
Correct Answer: 3. It generates a character from a Unicode code point.
Explanation: The chr
function in Python is used to generate a character from a Unicode code point.*
62. How can you open a file in Python in binary mode for both reading and writing?
Options:
file = open("example.txt", "rb+")
file = open("example.txt", "rwb")
file = open("example.txt", "bw")
file = open("example.txt", "br+")
Correct Answer:
file = open("example.txt", "rb+")
Explanation: To open a file in binary mode for both reading and writing, you can use the “rb+” mode.*
63. What is the purpose of the sorted
function in Python?
Options:
- It creates a sorted copy of a list.
- It sorts a list in place.
- It is used for sorting characters in a string.
- It returns the maximum value in an iterable.
Correct Answer:
- It creates a sorted copy of a list.
Explanation: The sorted
function in Python is used to create a new sorted list from the elements of an iterable.*
64. How can you convert a number to a string in Python?
Options:
number.to_string()
str(number)
string(number)
convert_to_string(number)
Correct Answer: 2. str(number)
Explanation: The str()
function in Python is used to convert a number to a string.*
65. What is the purpose of the enumerate
function in a loop in Python?
Options:
- It counts the number of elements in a list.
- It creates a new list.
- It returns the index and value of each element in an iterable.
- It is used for enumerating characters in a string.
Correct Answer: 3. It returns the index and value of each element in an iterable.
Explanation: The enumerate
function in Python is used in loops to iterate over both the index and the value of an iterable.*
66. How can you check if a string starts with a specific substring in Python?
Options:
string.startswith(substring)
substring.check_start(string)
start(string, substring)
string[0] == substring
Correct Answer:
string.startswith(substring)
Explanation: The startswith()
method in Python is used to check if a string starts with a specified prefix.*
67. What is the purpose of the next
function in Python?
Options:
- It generates the next number in a sequence.
- It is a reserved keyword for system-level operations.
- It returns the next item from an iterator.
- It creates a new instance of a class.
Correct Answer: 3. It returns the next item from an iterator.
Explanation: The next
function in Python is used to retrieve the next item from an iterator.*
68. How can you check if a variable is of a specific type in Python?
Options:
isinstance(variable, type)
variable.check_type(type)
variable.typeof(type)
type(variable) == type
Correct Answer:
isinstance(variable, type)
Explanation: The isinstance()
function in Python is used to check if a variable is an instance of a specific type.*
69. What is the purpose of the sum
function in Python?
Options:
- It calculates the sum of all elements in an iterable.
- It is used for bitwise sum operations.
- It concatenates strings in a list.
- It returns the maximum value in an iterable.
Correct Answer:
- It calculates the sum of all elements in an iterable.
Explanation: The sum
function in Python is used to calculate the sum of all elements in an iterable.*
70. How can you remove all occurrences of a specific element from a list in Python?
Options:
list.remove_all(element)
list.discard(element)
list.remove(element)
list.filter(element)
Correct Answer: 3. list.remove(element)
Explanation: The remove
method in Python is used to remove the first occurrence of a specified value from a list. To remove all occurrences, you may need to use a loop or list comprehension
71. What is the purpose of the isalnum
method in Python?
Options:
- It checks if a string contains only alphanumeric characters.
- It converts a string to lowercase.
- It checks if a string is empty.
- It removes all whitespace from a string.
Correct Answer:
- It checks if a string contains only alphanumeric characters.
Explanation: The isalnum
method in Python is used to check if a string contains only alphanumeric characters.*
72. How can you find the index of the first occurrence of a value in a list in Python?
Options:
list.index(value)
list.find(value)
find(list, value)
list.search(value)
Correct Answer:
list.index(value)
Explanation: The index
method in Python is used to find the index of the first occurrence of a specified value in a list.*
73. What is the purpose of the round
function in Python?
Options:
- It rounds a floating-point number to the nearest integer.
- It is used for rounding up a number.
- It is used for mathematical calculations.
- It rounds a number to a specified number of decimal places.
Correct Answer: 4. It rounds a number to a specified number of decimal places.
Explanation: The round
function in Python is used to round a number to a specified number of decimal places.*
74. How can you convert a list of strings to a single string with a delimiter in Python?
Options:
delimiter.join(list)
list.combine(delimiter)
str.concat(list, delimiter)
list + delimiter
Correct Answer:
delimiter.join(list)
Explanation: The join
method in Python is used to concatenate a list of strings into a single string, using a specified delimiter.*
75. What is the purpose of the strip
method in Python?
Options:
- It removes all whitespace characters from the beginning and end of a string.
- It splits a string into a list of substrings.
- It extracts a substring from a string.
- It is used for stripping comments from code.
Correct Answer:
- It removes all whitespace characters from the beginning and end of a string.
Explanation: The strip
method in Python is used to remove leading and trailing whitespace characters from a string.*
76. How can you convert a string to a list of words in Python?
Options:
list(string)
split(string)
string.to_list()
words(string)
Correct Answer: 2. split(string)
Explanation: The split
method in Python is used to split a string into a list of words, using whitespace as the default delimiter.*
77. What is the purpose of the bin
function in Python?
Options:
- It converts a number to a binary string.
- It is used for bitwise operations.
- It creates a binary file.
- It converts a binary string to an integer.
Correct Answer:
- It converts a number to a binary string.
Explanation: The bin
function in Python is used to convert an integer to a binary string.*
78. How can you check if a string ends with a specific suffix in Python?
Options:
string.ends(suffix)
string.endswith(suffix)
endswith(string, suffix)
suffix.check(string)
Correct Answer: 2. string.endswith(suffix)
Explanation: The endswith
method in Python is used to check if a string ends with a specified suffix.*
79. What is the purpose of the reversed
function in Python?
Options:
- It reverses the order of elements in a list.
- It creates a reversed copy of a string.
- It is used for reversing files.
- It generates a reverse iterator.
Correct Answer: 4. It generates a reverse iterator.
Explanation: The reversed
function in Python is used to create a reverse iterator, which can be used to iterate over the elements of a sequence in reverse order.*
80. How can you remove all whitespace characters from a string in Python?
Options:
string.trim()
strip(string)
string.remove_whitespace()
"".join(string.split())
Correct Answer: 4. "".join(string.split())
Explanation: One way to remove all whitespace characters from a string in Python is to use the split
method to create a list of words and then use "".join()
to concatenate the words without spaces.*
81. What is the purpose of the all
function in Python?
Options:
- It checks if any element in an iterable is true.
- It checks if all elements in an iterable are true.
- It returns the logical OR of all elements in an iterable.
- It is used for checking file permissions.
Correct Answer: 2. It checks if all elements in an iterable are true.
Explanation: The all
function in Python returns True
if all elements of an iterable are true, otherwise it returns False
.*
82. How can you check if a number is a prime number in Python?
Options:
isprime(number)
number.check_prime()
prime(number)
number % 2 == 0
Correct Answer:
isprime(number)
Explanation: Checking if a number is prime in Python often involves using a function like isprime()
that tests for divisibility by all numbers less than the square root of the given number.*
83. What is the purpose of the staticmethod
decorator in Python?
Options:
- It is used to define a static method in a class.
- It is a reserved keyword for system-level operations.
- It creates a new instance of a class.
- It is used for string formatting.
Correct Answer:
- It is used to define a static method in a class.
Explanation: The staticmethod
decorator in Python is used to define a static method in a class. A static method is a method that belongs to the class rather than an instance of the class.*
84. How can you concatenate two sets in Python?
Options:
set1.concat(set2)
set1 + set2
set1.extend(set2)
set1.update(set2)
Correct Answer: 4. set1.update(set2)
Explanation: The update
method in Python is used to add elements from another set (or any iterable) to an existing set, effectively concatenating them.*
85. What is the purpose of the min
function in Python?
Options:
- It finds the minimum value in a list.
- It is used for bitwise minimum operations.
- It creates a new minimum value.
- It returns the index of the minimum element in a list.
Correct Answer:
- It finds the minimum value in a list.
Explanation: The min
function in Python returns the smallest item in an iterable or the smallest of two or more arguments.*
86. How can you check if a string contains only numeric characters in Python?
Options:
string.isdigit()
string.isnumeric()
numeric(string)
check_numeric(string)
Correct Answer: 2. string.isnumeric()
Explanation: The isnumeric()
method in Python is used to check if all the characters in a string are numeric.*
87. What is the purpose of the divmod
function in Python?
Options:
- It divides two numbers and returns the quotient and remainder.
- It is used for bitwise division operations.
- It creates a new modulus value.
- It is used for dividing strings.
Correct Answer:
- It divides two numbers and returns the quotient and remainder.
Explanation: The divmod
function in Python takes two numbers and returns a pair of numbers (a tuple) consisting of their quotient and remainder when using integer division.*
88. How can you convert a dictionary to a list of key-value pairs in Python?
Options:
list(dictionary)
dictionary.to_list()
list(dictionary.items())
convert_to_list(dictionary)
Correct Answer: 3. list(dictionary.items())
Explanation: The items()
method in Python is used to return a list of key-value pairs as tuples. Using list()
on this result converts the dictionary to a list of tuples.*
89. What is the purpose of the filter
function in Python?
Options:
- It filters elements based on a given condition.
- It creates a new list containing the unique elements of an existing list.
- It applies a function to all the elements of an iterable.
- It is used for filtering files in a directory.
Correct Answer:
- It filters elements based on a given condition.
Explanation: The filter
function in Python is used to filter elements from an iterable based on a specified function or condition.*
90. How can you check if a list is empty in Python?
Options:
if list.is_empty():
if list == []:
if len(list) == 0:
if not list:
Correct Answer: 4. if not list:
Explanation: Checking if a list is empty in Python can be done using the not
keyword, as an empty list evaluates to False
in a boolean context.*
91. What is the purpose of the format
method in Python?
Options:
- It formats a string by replacing placeholders with values.
- It is used for formatting numbers.
- It creates a new format object.
- It formats a date and time.
Correct Answer:
- It formats a string by replacing placeholders with values.
Explanation: The format
method in Python is used to format a string by replacing placeholders with specified values.*
92. How can you convert a list of integers to a string in Python?
Options:
str(list)
"".join(map(str, list))
convert_to_string(list)
list.to_string()
Correct Answer: 2. "".join(map(str, list))
Explanation: Using map(str, list)
converts each integer in the list to a string, and then "".join()
concatenates them into a single string.*
93. What is the purpose of the callable
function in Python?
Options:
- It checks if an object is callable.
- It calls a function.
- It creates a callable object.
- It is used for making calls to external APIs.
Correct Answer:
- It checks if an object is callable.
Explanation: The callable
function in Python is used to check if an object appears to be callable (i.e., can be called as a function).*
94. How can you find the length of the longest word in a string in Python?
Options:
max(len(word) for word in string)
string.max_word_length()
len(max(string.split(), key=len))
longest_word_length(string)
Correct Answer: 3. len(max(string.split(), key=len))
Explanation: This expression splits the string into words using string.split()
, finds the word with the maximum length using max()
, and then calculates the length of that word.*
95. What is the purpose of the chr
function in Python?
Options:
- It calculates the absolute value of a number.
- It converts a character to its Unicode code point.
- It generates a character from a Unicode code point.
- It is used for character mapping.
Correct Answer: 3. It generates a character from a Unicode code point.
Explanation: The chr
function in Python is used to generate a character from a Unicode code point.*
96. How can you check if a string contains only alphabetic characters in Python?
Options:
string.isalpha()
string.isalphabetic()
is_alpha(string)
check_alphabetic(string)
Correct Answer:
string.isalpha()
Explanation: The isalpha
method in Python is used to check if all characters in a string are alphabetic.*
97. What is the purpose of the locals
function in Python?
Options:
- It is used for local variable declaration.
- It returns a dictionary of the current local symbol table.
- It creates local functions.
- It is used for localization of strings.
Correct Answer: 2. It returns a dictionary of the current local symbol table.
Explanation: The locals
function in Python returns a dictionary of the current local symbol table, which contains all local variables.*
98. How can you reverse the order of elements in a list in Python?
Options:
reversed(list)
list.reverse()
list.sort(reverse=True)
reverse(list)
Correct Answer:
reversed(list)
Explanation: The reversed
function in Python is used to reverse the order of elements in an iterable.*
99. What is the purpose of the len
function in Python?
Options:
- It calculates the length of a string.
- It is used for bitwise length operations.
- It returns the index of the maximum element in a list.
- It calculates the length of an iterable.
Correct Answer: 4. It calculates the length of an iterable.
Explanation: The len
function in Python is used to calculate the number of items in an iterable or the length of a string.*
100. How can you remove duplicate elements from a list in Python?
Options:
list.unique()
unique(list)
list.remove_duplicates()
list(set(list))
Correct Answer: 4. list(set(list))
Explanation: Converting the list to a set and then back to a list eliminates duplicate elements, as sets cannot contain duplicate items.*
Explanation: Converting the list to a set and then back to a list eliminates duplicate elements, as sets cannot contain duplicate items.*
101. What is the purpose of the eval
function in Python?
Options:
- It evaluates mathematical expressions.
- It evaluates logical expressions.
- It executes external commands.
- It evaluates the length of an iterable.
Correct Answer:
- It evaluates mathematical expressions.
Explanation: The eval
function in Python is used to evaluate mathematical expressions or other valid Python expressions from a string.*
102. How can you convert a list of strings to lowercase in Python?
Options:
list.lower()
lower(list)
[word.lower() for word in list]
convert_to_lowercase(list)
Correct Answer: 3. [word.lower() for word in list]
Explanation: List comprehension can be used to create a new list where each string is converted to lowercase using word.lower()
.*
103. What is the purpose of the bytearray
type in Python?
Options:
- It is used for representing boolean values.
- It represents a mutable sequence of bytes.
- It is used for bitwise operations.
- It is used for formatting strings.
Correct Answer: 2. It represents a mutable sequence of bytes.
Explanation: The bytearray
type in Python represents a mutable sequence of bytes, and it can be modified after creation.*
104. How can you get the current date and time in Python?
Options:
current_datetime()
datetime.now()
get_datetime()
now(datetime)
Correct Answer: 2. datetime.now()
Explanation: The datetime.now()
function in Python is used to get the current local date and time.*
105. What is the purpose of the sum
function in Python?
Options:
- It calculates the sum of all elements in an iterable.
- It is used for bitwise sum operations.
- It concatenates strings in a list.
- It returns the maximum value in an iterable.
Correct Answer:
- It calculates the sum of all elements in an iterable.
Explanation: The sum
function in Python is used to calculate the sum of all elements in an iterable.*
106. How can you find the square root of a number in Python?
Options:
number.sqrt()
sqrt(number)
math.square_root(number)
number ** 0.5
Correct Answer: 4. number ** 0.5
Explanation: Calculating the square root of a number in Python can be done using the exponentiation operator **
with the value 0.5
.*
107. What is the purpose of the ord
function in Python?
Options:
- It calculates the absolute value of a number.
- It converts a character to its Unicode code point.
- It generates a character from a Unicode code point.
- It is used for character mapping.
Correct Answer: 2. It converts a character to its Unicode code point.
Explanation: The ord
function in Python is used to get the Unicode code point of a character.*
108. How can you check if a number is a power of two in Python?
Options:
number.power_of_two()
power_of_two(number)
number % 2 == 0
(number & (number - 1)) == 0
Correct Answer: 4. (number & (number - 1)) == 0
Explanation: Checking if a number is a power of two in Python can be done using bitwise operations.*
109. What is the purpose of the bin
function in Python?
Options:
- It converts a number to a binary string.
- It is used for bitwise operations.
- It creates a binary file.
- It converts a binary string to an integer.
Correct Answer:
- It converts a number to a binary string.
Explanation: The bin
function in Python is used to convert an integer to a binary string.*
110. How can you concatenate two dictionaries in Python?
Options:
dict1.concat(dict2)
dict1 + dict2
dict1.extend(dict2)
dict1.update(dict2)
Correct Answer: 4. dict1.update(dict2)
Explanation: The update
method in Python is used to add key-value pairs from another dictionary to an existing dictionary, effectively concatenating them.*
111. What is the purpose of the locals
function in Python?
Options:
- It is used for local variable declaration.
- It returns a dictionary of the current local symbol table.
- It creates local functions.
- It is used for localization of strings.
Correct Answer: 2. It returns a dictionary of the current local symbol table.
Explanation: The locals
function in Python returns a dictionary of the current local symbol table, which contains all local variables.*
112. How can you check if a string contains only alphabetic characters in Python?
Options:
string.isalpha()
string.isalphabetic()
is_alpha(string)
check_alphabetic(string)
Correct Answer:
string.isalpha()
Explanation: The isalpha
method in Python is used to check if all characters in a string are alphabetic.*
113. What is the purpose of the reversed
function in Python?
Options:
- It reverses the order of elements in a list.
- It creates a reversed copy of a string.
- It is used for reversing files.
- It generates a reverse iterator.
Correct Answer: 4. It generates a reverse iterator.
Explanation: The reversed
function in Python is used to create a reverse iterator, which can be used to iterate over the elements of a sequence in reverse order.*
114. How can you check if a variable is of a specific type in Python?
Options:
isinstance(variable, type)
variable.check_type(type)
variable.typeof(type)
type(variable) == type
Correct Answer:
isinstance(variable, type)
Explanation: The isinstance()
function in Python is used to check if a variable is an instance of a specific type.*
115. What is the purpose of the filter
function in Python?
Options:
- It filters elements based on a given condition.
- It creates a new list containing the unique elements of an existing list.
- It applies a function to all the elements of an iterable.
- It is used for filtering files in a directory.
Correct Answer:
- It filters elements based on a given condition.
Explanation: The filter
function in Python is used to filter elements from an iterable based on a specified function or condition.*
116. How can you convert a number to a string in Python?
Options:
number.to_string()
str(number)
string(number)
convert_to_string(number)
Correct Answer: 2. str(number)
Explanation: The str()
function in Python is used to convert a number to a string.*
117. What is the purpose of the bin
function in Python?
Options:
- It converts a number to a binary string.
- It is used for bitwise operations.
- It creates a binary file.
- It converts a binary string to an integer.
Correct Answer:
- It converts a number to a binary string.
Explanation: The bin
function in Python is used to convert an integer to a binary string.*
118. How can you check if a string ends with a specific suffix in Python?
Options:
string.ends(suffix)
string.endswith(suffix)
endswith(string, suffix)
suffix.check(string)
Correct Answer: 2. string.endswith(suffix)
Explanation: The endswith
method in Python is used to check if a string ends with a specified suffix.*
119. What is the purpose of the any
function in Python?
Options:
- It checks if all elements in an iterable are true.
- It checks if any element in an iterable is true.
- It returns the logical AND of all elements in an iterable.
- It is used for logical OR operations.
Correct Answer: 2. It checks if any element in an iterable is true.
Explanation: The any
function in Python returns True
if at least one element of an iterable is true, otherwise it returns False
.*
120. How can you remove an element from a set in Python?
Options:
set.remove(element)
set.delete(element)
set.discard(element)
remove(set, element)
Correct Answer: 3. set.discard(element)
Explanation: The discard()
method in Python is used to remove a specified element from a set, if it is present.*
121. What is the purpose of the round
function in Python?
Options:
- It rounds a floating-point number to the nearest integer.
- It is used for rounding up a number.
- It is used for mathematical calculations.
- It rounds a number to a specified number of decimal places.
Correct Answer: 4. It rounds a number to a specified number of decimal places.
Explanation: The round
function in Python is used to round a number to a specified number of decimal places.*
122. How can you check if a string is a palindrome in Python?
Options:
string.is_palindrome()
is_palindrome(string)
string == reverse(string)
string == string[::-1]
Correct Answer: 4. string == string[::-1]
Explanation: Checking if a string is a palindrome in Python can be done by comparing the original string with its reverse.*
123. What is the purpose of the enumerate
function in Python?
Options:
- It counts the number of elements in a list.
- It creates a new list.
- It returns the index and value of each element in an iterable.
- It is used for enumerating characters in a string.
Correct Answer: 3. It returns the index and value of each element in an iterable.
Explanation: The enumerate
function in Python is used in loops to iterate over both the index and the value of an iterable.*
124. How can you convert a list of integers to a list of strings in Python?
Options:
[str(list)]
convert_to_strings(list)
[str(x) for x in list]
list.to_strings()
Correct Answer: 3. [str(x) for x in list]
Explanation: List comprehension can be used to create a new list where each integer is converted to a string using str(x)
.*
125. What is the purpose of the map
function in Python?
Options:
- It is used for creating maps.
- It applies a function to all the elements of an iterable.
- It generates a map object.
- It creates a dictionary.
Correct Answer: 2. It applies a function to all the elements of an iterable.
Explanation: The map
function in Python applies a specified function to all items in an input iterable (list, tuple, etc.) and returns an iterator that produces the results.*
126. How can you check if a number is even in Python?
Options:
number.is_even()
is_even(number)
number % 2 == 0
even(number)
Correct Answer: 3. number % 2 == 0
Explanation: Checking if a number is even in Python can be done using the modulus operator %
. If the result is 0, the number is even.*
127. What is the purpose of the chr
function in Python?
Options:
- It calculates the absolute value of a number.
- It converts a character to its Unicode code point.
- It generates a character from a Unicode code point.
- It is used for character mapping.
Correct Answer: 3. It generates a character from a Unicode code point.
Explanation: The chr
function in Python is used to generate a character from a Unicode code point.*
128. How can you find the index of the last occurrence of a value in a list in Python?
Options:
list.find_last(value)
list.rfind(value)
list.index_last(value)
list.search_last(value)
Correct Answer: 2. list.rfind(value)
Explanation: The rfind
method in Python is used to find the index of the last occurrence of a specified value in a list.*
129. What is the purpose of the len
function in Python?
Options:
- It calculates the length of a string.
- It is used for bitwise length operations.
- It returns the index of the maximum element in a list.
- It calculates the length of an iterable.
Correct Answer: 4. It calculates the length of an iterable.
Explanation: The len
function in Python is used to calculate the number of items in an iterable or the length of a string.*
130. How can you check if a list is a subset of another list in Python?
Options:
list.is_subset(other_list)
subset(list, other_list)
set(list).issubset(set(other_list))
list.check_subset(other_list)
Correct Answer: 3. set(list).issubset(set(other_list))
Explanation: One way to check if a list is a subset of another list in Python is to convert both lists to sets and then use the issubset
method.*
Explanation: One way to check if a list is a subset of another list in Python is to convert both lists to sets and then use the issubset
method.*
141. What is the purpose of the isinstance
function in Python?
Options:
- It checks if an object is an instance of a specific class or type.
- It creates a new instance of a class.
- It checks if an object is empty.
- It is used for instantiating classes.
Correct Answer:
- It checks if an object is an instance of a specific class or type.
Explanation: The isinstance
function in Python is used to check if an object is an instance of a specified class or type.*
142. How can you reverse the order of characters in a string in Python?
Options:
string.reverse()
reverse(string)
string[::-1]
string.invert()
Correct Answer: 3. string[::-1]
Explanation: Using the slicing syntax [::-1]
in Python reverses the order of characters in a string.*
143. What is the purpose of the list
function in Python?
Options:
- It creates a new list.
- It converts an iterable to a list.
- It checks if an object is a list.
- It is used for list manipulation.
Correct Answer: 2. It converts an iterable to a list.
Explanation: The list
function in Python is used to convert an iterable (e.g., tuple, string) to a list.*
144. How can you check if a list is not empty in Python?
Options:
if not list:
if list.not_empty():
if list != []:
if len(list) > 0:
Correct Answer:
if not list:
Explanation: Checking if a list is not empty in Python can be done using the not
keyword, as an empty list evaluates to False
in a boolean context.*
145. What is the purpose of the ord
function in Python?
Options:
- It calculates the absolute value of a number.
- It converts a character to its Unicode code point.
- It generates a character from a Unicode code point.
- It is used for character mapping.
Correct Answer: 2. It converts a character to its Unicode code point.
Explanation: The ord
function in Python is used to get the Unicode code point of a character.*
146. How can you check if a number is positive in Python?
Options:
number.positive()
positive(number)
number > 0
is_positive(number)
Correct Answer: 3. number > 0
Explanation: Checking if a number is positive in Python can be done by comparing it to zero using the >
operator.*
147. What is the purpose of the set
function in Python?
Options:
- It creates a new set.
- It converts an iterable to a set.
- It checks if an object is a set.
- It is used for set operations.
Correct Answer: 2. It converts an iterable to a set.
Explanation: The set
function in Python is used to convert an iterable (e.g., list, tuple) to a set.*
148. How can you check if a string starts with a specific prefix in Python?
Options:
string.starts(prefix)
string.startswith(prefix)
startswith(string, prefix)
prefix.check(string)
Correct Answer: 2. string.startswith(prefix)
Explanation: The startswith
method in Python is used to check if a string starts with a specified prefix.*
149. What is the purpose of the max
function in Python?
Options:
- It finds the maximum value in a list.
- It is used for bitwise maximum operations.
- It creates a new maximum value.
- It returns the index of the maximum element in a list.
Correct Answer:
- It finds the maximum value in a list.
Explanation: The max
function in Python returns the largest item in an iterable or the largest of two or more arguments.*
150. How can you check if a number is a perfect square in Python?
Options:
number.perfect_square()
perfect_square(number)
number == int(number**0.5)**2
(number & (number - 1)) == 0
Correct Answer: 3. number == int(number**0.5)**2
Explanation: Checking if a number is a perfect square in Python can be done by comparing it to the square of its integer square root.*
151. What is the purpose of the abs
function in Python?
Options:
- It converts a value to an absolute value.
- It is used for abstract mathematical operations.
- It calculates the absolute sum of elements in an iterable.
- It is used for abstract data structures.
Correct Answer:
- It converts a value to an absolute value.
Explanation: The abs
function in Python is used to return the absolute value of a number.*
152. How can you convert a string to an integer in Python?
Options:
int(string)
string.to_int()
integer(string)
convert_to_int(string)
Correct Answer:
int(string)
Explanation: The int
function in Python is used to convert a string or a number to an integer.*
153. What is the purpose of the any
function in Python?
Options:
- It checks if all elements in an iterable are true.
- It checks if any element in an iterable is true.
- It returns the logical AND of all elements in an iterable.
- It is used for logical OR operations.
Correct Answer: 2. It checks if any element in an iterable is true.
Explanation: The any
function in Python returns True
if at least one element of an iterable is true, otherwise it returns False
.*
154. How can you find the index of the maximum element in a list in Python?
Options:
list.find_max()
list.index(max(list))
max_index(list)
list.max_index()
Correct Answer: 2. list.index(max(list))
Explanation: The index
method in Python is used to find the index of the first occurrence of a specified value in a list.*
155. What is the purpose of the chr
function in Python?
Options:
- It calculates the absolute value of a number.
- It converts a character to its Unicode code point.
- It generates a character from a Unicode code point.
- It is used for character mapping.
Correct Answer: 3. It generates a character from a Unicode code point.
Explanation: The chr
function in Python is used to generate a character from a Unicode code point.*
156. How can you check if a string is empty in Python?
Options:
if not string:
string.is_empty()
if string == ''
string.check_empty()
Correct Answer:
if not string:
Explanation: Checking if a string is empty in Python can be done using the not
keyword, as an empty string evaluates to False
in a boolean context.*
157. What is the purpose of the filter
function in Python?
Options:
- It filters elements based on a given condition.
- It creates a new list containing the unique elements of an existing list.
- It applies a function to all the elements of an iterable.
- It is used for filtering files in a directory.
Correct Answer:
- It filters elements based on a given condition.
Explanation: The filter
function in Python is used to filter elements from an iterable based on a specified function or condition.*
158. How can you find the index of the minimum element in a list in Python?
Options:
min_index(list)
list.index(min(list))
list.find_min()
list.min_index()
Correct Answer: 2. list.index(min(list))
Explanation: The index
method in Python is used to find the index of the first occurrence of a specified value in a list.*
159. What is the purpose of the sum
function in Python?
Options:
- It calculates the sum of all elements in an iterable.
- It is used for bitwise sum operations.
- It concatenates strings in a list.
- It returns the maximum value in an iterable.
Correct Answer:
- It calculates the sum of all elements in an iterable.
Explanation: The sum
function in Python is used to calculate the sum of all elements in an iterable.*
160. How can you check if a string contains only numeric characters in Python?
Options:
string.isnumeric()
string.isnumericchars()
is_numeric(string)
check_numeric(string)
Correct Answer:
string.isnumeric()
Explanation: The isnumeric
method in Python is used to check if all characters in a string are numeric.*
161. What is the purpose of the round
function in Python?
Options:
- It rounds a floating-point number to the nearest integer.
- It is used for rounding up a number.
- It is used for mathematical calculations.
- It rounds a number to a specified number of decimal places.
Correct Answer: 4. It rounds a number to a specified number of decimal places.
Explanation: The round
function in Python is used to round a number to a specified number of decimal places.*
162. How can you check if a list contains duplicates in Python?
Options:
list.has_duplicates()
has_duplicates(list)
len(set(list)) != len(list)
list.contains_duplicates()
Correct Answer: 3. len(set(list)) != len(list)
Explanation: Checking for duplicates in a list in Python can be done by converting the list to a set and comparing the lengths.*
163. What is the purpose of the sorted
function in Python?
Options:
- It sorts elements in a list in ascending order.
- It creates a sorted copy of a set.
- It is used for sorting files in a directory.
- It sorts characters in a string.
Correct Answer:
- It sorts elements in a list in ascending order.
Explanation: The sorted
function in Python is used to sort the elements of an iterable in ascending order.*
164. How can you check if a list is a palindrome in Python?
Options:
list.is_palindrome()
is_palindrome(list)
list == list[::-1]
list.check_palindrome()
Correct Answer: 3. list == list[::-1]
Explanation: Checking if a list is a palindrome in Python can be done by comparing the original list with its reverse.*
165. What is the purpose of the tuple
function in Python?
Options:
- It creates a new tuple.
- It converts an iterable to a tuple.
- It checks if an object is a tuple.
- It is used for tuple operations.
Correct Answer: 2. It converts an iterable to a tuple.
Explanation: The tuple
function in Python is used to convert an iterable (e.g., list, string) to a tuple.*
166. How can you check if a string is a valid identifier in Python?
Options:
string.isidentifier()
is_identifier(string)
check_identifier(string)
identifier(string)
Correct Answer:
string.isidentifier()
Explanation: The isidentifier
method in Python is used to check if a string is a valid identifier.*
167. What is the purpose of the min
function in Python?
Options:
- It finds the minimum value in a list.
- It is used for bitwise minimum operations.
- It creates a new minimum value.
- It returns the index of the minimum element in a list.
Correct Answer:
- It finds the minimum value in a list.
Explanation: The min
function in Python returns the smallest item in an iterable or the smallest of two or more arguments.*
168. How can you check if a string contains only whitespace characters in Python?
Options:
string.iswhitespace()
string.isspace()
is_whitespace(string)
check_whitespace(string)
Correct Answer: 2. string.isspace()
Explanation: The isspace
method in Python is used to check if all characters in a string are whitespace characters.*
169. What is the purpose of the len
function in Python?
Options:
- It calculates the length of a string.
- It is used for bitwise length operations.
- It returns the index of the maximum element in a list.
- It calculates the length of an iterable.
Correct Answer: 4. It calculates the length of an iterable.
Explanation: The len
function in Python is used to calculate the number of items in an iterable or the length of a string.*
170. How can you convert a string to uppercase in Python?
Options:
string.upper()
uppercase(string)
convert_to_upper(string)
string.to_uppercase()
Correct Answer:
string.upper()
Explanation: The upper
method in Python is used to convert all characters in a string to uppercase.*
171. What is the purpose of the ord
function in Python?
Options:
- It calculates the absolute value of a number.
- It converts a character to its Unicode code point.
- It generates a character from a Unicode code point.
- It is used for character mapping.
Correct Answer: 2. It converts a character to its Unicode code point.
Explanation: The ord
function in Python is used to get the Unicode code point of a character.*
172. How can you check if a string ends with a specific suffix in Python?
Options:
string.ends(suffix)
string.endswith(suffix)
endswith(string, suffix)
suffix.check(string)
Correct Answer: 2. string.endswith(suffix)
Explanation: The endswith
method in Python is used to check if a string ends with a specified suffix.*
173. What is the purpose of the sum
function in Python?
Options:
- It calculates the sum of all elements in an iterable.
- It is used for bitwise sum operations.
- It concatenates strings in a list.
- It returns the maximum value in an iterable.
Correct Answer:
- It calculates the sum of all elements in an iterable.
Explanation: The sum
function in Python is used to calculate the sum of all elements in an iterable.*
174. How can you remove leading and trailing whitespace from a string in Python?
Options:
trim(string)
string.trim()
string.strip()
remove_whitespace(string)
Correct Answer: 3. string.strip()
Explanation: The strip
method in Python is used to remove leading and trailing whitespace from a string.*
175. What is the purpose of the map
function in Python?
Options:
- It is used for creating maps.
- It applies a function to all the elements of an iterable.
- It generates a map object.
- It creates a dictionary.
Correct Answer: 2. It applies a function to all the elements of an iterable.
Explanation: The map
function in Python applies a specified function to all items in an input iterable (list, tuple, etc.) and returns an iterator that produces the results.*
176. How can you concatenate two lists in Python?
Options:
list.concat(other_list)
list + other_list
concat(list, other_list)
list.combine(other_list)
Correct Answer: 2. list + other_list
Explanation: The +
operator in Python is used for concatenating two lists.*
177. What is the purpose of the float
function in Python?
Options:
- It is used for floating-point arithmetic.
- It converts a number to a floating-point number.
- It creates a new float object.
- It is used for floating-point comparison.
Correct Answer: 2. It converts a number to a floating-point number.
Explanation: The float
function in Python is used to convert a number or a string containing a number to a floating-point number.*
178. How can you check if a number is negative in Python?
Options:
number < 0
is_negative(number)
number.is_negative()
negative(number)
Correct Answer:
number < 0
Explanation: Checking if a number is negative in Python can be done by comparing it to zero using the <
operator.*
179. What is the purpose of the zip
function in Python?
Options:
- It compresses files.
- It creates a ZIP archive.
- It combines two or more iterables element-wise.
- It is used for zipping folders.
Correct Answer: 3. It combines two or more iterables element-wise.
Explanation: The zip
function in Python is used to combine elements from two or more iterables (e.g., lists, tuples) element-wise.*
180. How can you convert a list of strings to a single string in Python?
Options:
' '.join(list)
list.to_string()
convert_to_string(list)
string(list)
Correct Answer:
' '.join(list)
Explanation: The join
method in Python is used to concatenate a list of strings into a single string.*
181. What is the purpose of the isalpha
method in Python?
Options:
- It checks if a string contains only alphabetic characters.
- It converts a string to lowercase.
- It checks if a string is empty.
- It removes all whitespace from a string.
Correct Answer:
- It checks if a string contains only alphabetic characters.
Explanation: The isalpha
method in Python is used to check if all characters in a string are alphabetic.*
182. How can you find the index of the first occurrence of a substring in a string in Python?
Options:
string.find(substring)
string.index(substring)
substring.first_index(string)
find(string, substring)
Correct Answer:
string.find(substring)
Explanation: The find
method in Python is used to find the index of the first occurrence of a specified substring in a string.*
183. What is the purpose of the enumerate
function in Python?
Options:
- It counts the number of elements in a list.
- It creates a new list.
- It returns the index and value of each element in an iterable.
- It is used for enumerating characters in a string.
Correct Answer: 3. It returns the index and value of each element in an iterable.
Explanation: The enumerate
function in Python is used in loops to iterate over both the index and the value of an iterable.*
184. How can you check if a list is a subset of another list in Python?
Options:
list.is_subset(other_list)
subset(list, other_list)
set(list).issubset(set(other_list))
list.check_subset(other_list)
Correct Answer: 3. set(list).issubset(set(other_list))
Explanation: One way to check if a list is a subset of another list in Python is to convert both lists to sets and then use the issubset
method.*
185. What is the purpose of the chr
function in Python?
Options:
- It calculates the absolute value of a number.
- It converts a character to its Unicode code point.
- It generates a character from a Unicode code point.
- It is used for character mapping.
Correct Answer: 3. It generates a character from a Unicode code point.
Explanation: The chr
function in Python is used to generate a character from a Unicode code point.*
186. How can you check if a value is present in a list in Python?
Options:
value.check(list)
list.contains(value)
value in list
list.check_value(value)
Correct Answer: 3. value in list
Explanation: The in
keyword in Python is used to check if a value is present in a list.*
187. What is the purpose of the isdigit
method in Python?
Options:
- It checks if a string is composed of digits only.
- It converts a string to an integer.
- It is used for string formatting.
- It checks if a string contains only alphanumeric characters.
Correct Answer:
- It checks if a string is composed of digits only.
Explanation: The isdigit
method in Python is used to check if a string consists of digits only.*
188. How can you convert a list of integers to a list of strings in Python?
Options:
[str(list)]
convert_to_strings(list)
[str(x) for x in list]
list.to_strings()
Correct Answer: 3. [str(x) for x in list]
Explanation: List comprehension can be used to create a new list where each integer is converted to a string using str(x)
.*
189. What is the purpose of the map
function in Python?
Options:
- It is used for creating maps.
- It applies a function to all the elements of an iterable.
- It generates a map object.
- It creates a dictionary.
Correct Answer: 2. It applies a function to all the elements of an iterable.
Explanation: The map
function in Python applies a specified function to all items in an input iterable (list, tuple, etc.) and returns an iterator that produces the results.*
190. How can you check if a number is even in Python?
Options:
number.is_even()
is_even(number)
number % 2 == 0
even(number)
Correct Answer: 3. number % 2 == 0
Explanation: Checking if a number is even in Python can be done using the modulus operator %
. If the result is 0, the number is even.*
Explanation: Checking if a number is even in Python can be done using the modulus operator %
. If the result is 0, the number is even.*