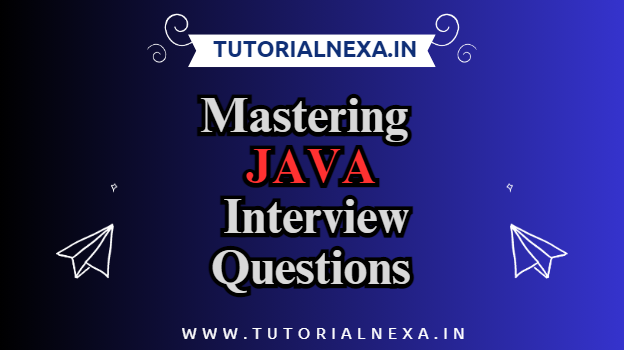
1. What is the default value of a boolean variable in Java?
a. 0
b. 1
c. false
d. true
Correct Answer: c. false
Explanation: In Java, the default value of a boolean variable is false
.
2. Which keyword is used to declare a constant in Java?
a. const
b. final
c. static
d. constant
Correct Answer: b. final
Explanation: The final
keyword is used in Java to declare a constant.
3. What is the main purpose of the “this” keyword in Java?
a. Referencing the current object
b. Declaring a new object
c. Accessing superclass members
d. Specifying method arguments
Correct Answer: a. Referencing the current object
Explanation: The “this” keyword in Java is used to refer to the current instance of the class.
4. How is memory allocated for objects in Java?
a. Stack
b. Heap
c. Queue
d. Array
Correct Answer: b. Heap
Explanation: In Java, objects are allocated memory in the heap.
5. Which of the following is a marker interface in Java?
a. Serializable
b. Cloneable
c. RandomAccess
d. All of the above
Correct Answer: d. All of the above
Explanation: Serializable, Cloneable, and RandomAccess are marker interfaces in Java.
6. What is the purpose of the “super” keyword in Java?
a. Invoking the superclass method
b. Accessing the superclass variable
c. Referring to the current object
d. Creating a new object
Correct Answer: a. Invoking the superclass method
Explanation: The “super” keyword in Java is used to invoke the method of the superclass.
7. What is the return type of the “main” method in Java?
a. void
b. int
c. String
d. double
Correct Answer: a. void
Explanation: The “main” method in Java has a return type of void.
8. Which collection class in Java uses a dynamic array to store elements?
a. ArrayList
b. LinkedList
c. HashSet
d. TreeMap
Correct Answer: a. ArrayList
Explanation: ArrayList in Java uses a dynamic array to store elements.
9. What is the purpose of the “try,” “catch,” and “finally” blocks in exception handling?
a. “try” is for catching exceptions, “catch” is for handling normal flow, and “finally” is for cleanup.
b. “try” is for normal flow, “catch” is for catching exceptions, and “finally” is optional.
c. “try” is optional, “catch” is for normal flow, and “finally” is for catching exceptions.
d. “try” is for cleanup, “catch” is for normal flow, and “finally” is optional.
Correct Answer: a. “try” is for catching exceptions, “catch” is for handling normal flow, and “finally” is for cleanup.
Explanation: The “try” block contains the code that may throw exceptions, “catch” handles the exception, and “finally” is used for cleanup code that must be executed regardless of whether an exception is thrown or not.
10. What is the difference between “==” and “.equals()” when comparing two objects in Java?
a. They are interchangeable; there is no difference.
b. “==” compares the references, while “.equals()” compares the content.
c. “==” compares the content, while “.equals()” compares the references.
d. Both are used for reference comparison.
Correct Answer: b. “==” compares the references, while “.equals()” compares the content.
Explanation: In Java, “==” checks if two references point to the same object, while “.equals()” is used to compare the content (implementation-dependent and needs to be overridden in the class).
11. What is the purpose of the “static” keyword in Java?
a. Making a variable or method applicable to the class rather than an instance.
b. Dynamically changing the type of a variable.
c. Allowing variables to be modified at runtime.
d. Enforcing encapsulation.
Correct Answer: a. Making a variable or method applicable to the class rather than an instance.
Explanation: The “static” keyword in Java is used to create variables and methods that belong to the class, not to the instance of the class.
12. Which of the following statements about interfaces in Java is true?
a. An interface can have constructors.
b. An interface can extend multiple interfaces.
c. An interface can have non-public methods.
d. An interface can contain instance variables.
Correct Answer: b. An interface can extend multiple interfaces.
Explanation: In Java, an interface can extend multiple interfaces to achieve multiple inheritances of method signatures.
13. What is the purpose of the “break” statement in a loop in Java?
a. To terminate the loop and exit the program.
b. To skip the current iteration and move to the next one.
c. To execute the code in the loop indefinitely.
d. To jump to a specific label within the loop.
Correct Answer: b. To skip the current iteration and move to the next one.
Explanation: The “break” statement in Java is used to terminate the loop prematurely and move to the next iteration.
14. Which of the following is true about the “StringBuilder” class in Java?
a. It is an immutable class.
b. It is synchronized, making it thread-safe.
c. It is more memory-efficient than “String” for concatenating multiple strings.
d. It does not have a “toString()” method.
Correct Answer: c. It is more memory-efficient than “String” for concatenating multiple strings.
Explanation: “StringBuilder” is mutable and more memory-efficient than “String” when performing multiple concatenations.
15. What is the purpose of the “transient” keyword in Java?
a. To indicate that a variable should not be serialized.
b. To specify that a variable should be initialized with a default value.
c. To make a variable accessible across different classes.
d. To declare a variable constant.
Correct Answer: a. To indicate that a variable should not be serialized.
Explanation: The “transient” keyword in Java is used to indicate that a variable should not be included in the object’s serialized form.
16. In Java, what is the difference between an abstract class and an interface?
a. An abstract class can have constructors, while an interface cannot.
b. An abstract class can have multiple inheritance, while an interface cannot.
c. An abstract class can have both abstract and non-abstract methods, while an interface can only have abstract methods.
d. An abstract class can be instantiated, while an interface cannot.
Correct Answer: c. An abstract class can have both abstract and non-abstract methods, while an interface can only have abstract methods.
Explanation: Abstract classes in Java can have both abstract and non-abstract methods, while interfaces can only have abstract methods (methods without a body).
17. What is the purpose of the “instanceof” operator in Java?
a. To check if an object is of a particular type at runtime.
b. To create a new instance of a class.
c. To compare two objects for equality.
d. To convert one type of object to another.
Correct Answer: a. To check if an object is of a particular type at runtime.
Explanation: The “instanceof” operator in Java is used to test whether an object is an instance of a particular class or interface at runtime.
18. How is an abstract method in an abstract class different from a regular method in a concrete class?
a. Abstract methods must have a body, while regular methods do not.
b. Abstract methods are declared using the “abstract” keyword and do not have a body, while regular methods have both declaration and implementation.
c. Regular methods cannot be overridden in subclasses, while abstract methods can.
d. Abstract methods can only be defined in interfaces, not in abstract classes.
Correct Answer: b. Abstract methods are declared using the “abstract” keyword and do not have a body, while regular methods have both declaration and implementation.
Explanation: Abstract methods in abstract classes are declared using the “abstract” keyword and do not provide an implementation in the abstract class, leaving it to be implemented by subclasses.
19. What is the purpose of the “finally” block in a try-catch-finally statement in Java?
a. To catch and handle exceptions.
b. To execute code only when there is an exception.
c. To define code that must be executed whether an exception is thrown or not.
d. To terminate the program.
Correct Answer: c. To define code that must be executed whether an exception is thrown or not.
Explanation: The “finally” block in a try-catch-finally statement is used to define code that will be executed regardless of whether an exception is thrown or not.
20. Which of the following Java collections is synchronized and thread-safe?
a. ArrayList
b. HashSet
c. Vector
d. TreeMap
Correct Answer: c. Vector
Explanation: Vector
in Java is synchronized, which means it is thread-safe. However, note that newer collections classes like ArrayList
are not synchronized by default.
21. What is the purpose of the “super()” keyword in a constructor in Java?
a. To call the superclass method.
b. To instantiate the superclass.
c. To call the constructor of the current class.
d. To initialize the superclass variables.
Correct Answer: b. To instantiate the superclass.
Explanation: The “super()” keyword in a constructor is used to call the constructor of the superclass and instantiate the superclass.
22. What is the purpose of the finalize()
method in Java?
a. To force garbage collection for the object.
b. To indicate that an object is ready for destruction.
c. To release system resources before an object is reclaimed by the garbage collector.
d. To prevent an object from being collected by the garbage collector.
Correct Answer: c. To release system resources before an object is reclaimed by the garbage collector.
Explanation: The finalize()
method in Java is called by the garbage collector before it reclaims the memory occupied by an object. It is often used to release system resources held by the object.
23. In Java, what is the purpose of the static
block?
a. To define static variables in a class.
b. To initialize non-static variables.
c. To execute code when the class is loaded into the JVM.
d. To create an instance of the class.
Correct Answer: c. To execute code when the class is loaded into the JVM.
Explanation: The static
block in Java is used to execute code when the class is loaded, and it is executed only once.
24. What is the purpose of the compareTo()
method in the Comparable
interface in Java?
a. To compare two objects for equality.
b. To compare two objects based on their content.
c. To compare the memory addresses of two objects.
d. To provide a total ordering of objects that implement the interface.
Correct Answer: d. To provide a total ordering of objects that implement the interface.
Explanation: The compareTo()
method in the Comparable
interface is used to provide a total ordering of objects. It returns a negative integer, zero, or a positive integer depending on whether the current object is less than, equal to, or greater than the specified object.
25. What is the purpose of the equals()
method in Java?
a. To compare the memory addresses of two objects.
b. To compare two objects for equality based on their content.
c. To determine if an object is an instance of a particular class.
d. To check if an object is null.
Correct Answer: b. To compare two objects for equality based on their content.
Explanation: The equals()
method in Java is typically overridden to compare the content of objects for equality.
26. What is the significance of the volatile
keyword in Java?
a. It ensures that a variable is not modified by multiple threads.
b. It guarantees atomicity for operations on the variable.
c. It prevents the variable from being cached by threads.
d. It indicates that a variable may be changed by multiple threads.
Correct Answer: d. It indicates that a variable may be changed by multiple threads.
Explanation: The volatile
keyword in Java is used to indicate that a variable’s value may be changed by multiple threads.
27. In Java, what is the purpose of the break
statement within a switch statement?
a. To exit the entire program.
b. To terminate the current iteration of the loop.
c. To exit the switch statement and resume normal program flow.
d. To skip the next case in the switch statement.
Correct Answer: c. To exit the switch statement and resume normal program flow.
Explanation: The break
statement within a switch statement is used to exit the switch and continue with the next statement after the switch.
28. How is an interface in Java different from an abstract class?
a. An interface can have constructors, while an abstract class cannot.
b. An abstract class can have both abstract and non-abstract methods, while an interface can only have abstract methods.
c. An interface can have variables, while an abstract class cannot.
d. An abstract class cannot be implemented by multiple classes, while an interface can.
Correct Answer: b. An abstract class can have both abstract and non-abstract methods, while an interface can only have abstract methods.
Explanation: Abstract classes in Java can have both abstract and non-abstract methods, while interfaces can only have abstract methods.
29. What is the purpose of the Math.random()
method in Java?
a. To generate a random boolean value.
b. To generate a random integer value.
c. To generate a random floating-point value between 0.0 (inclusive) and 1.0 (exclusive).
d. To generate a random number with a specified range.
Correct Answer: c. To generate a random floating-point value between 0.0 (inclusive) and 1.0 (exclusive).
Explanation: Math.random()
in Java generates a random double value between 0.0 (inclusive) and 1.0 (exclusive).
30. What is the purpose of the super()
constructor in a subclass in Java?
a. To call the constructor of the superclass.
b. To instantiate the subclass.
c. To reference the superclass variable.
d. To create an instance of the superclass.
Correct Answer: a. To call the constructor of the superclass.
Explanation: The super()
constructor in Java is used to call the constructor of the superclass from the constructor of the subclass.
31. How can you achieve multiple inheritances in Java?
a. By using the extends
keyword for classes and interfaces.
b. By using the implements
keyword for interfaces.
c. Java does not support multiple inheritances.
d. By using the inherits
keyword for classes.
Correct Answer: c. Java does not support multiple inheritances.
Explanation: Java supports multiple inheritances through interfaces, but a class can only extend one class.
32. What is the purpose of the try-with-resources
statement in Java?
a. To catch and handle exceptions in a concise manner.
b. To create a try-catch block for critical sections of code.
c. To ensure that resources are closed after being used, automatically.
d. To specify the scope of a try block.
Correct Answer: c. To ensure that resources are closed after being used, automatically.
Explanation: The try-with-resources
statement in Java is used for automatic resource management, ensuring that resources like files or sockets are closed after the try block is executed.
33. In Java, what is the difference between the ==
operator and the equals()
method for comparing objects?
a. They are interchangeable; there is no difference.
b. ==
compares the memory addresses of objects, while equals()
compares their content.
c. ==
compares the content of objects, while equals()
compares the memory addresses.
d. Both are used for reference comparison.
Correct Answer: b. ==
compares the memory addresses of objects, while equals()
compares their content.
Explanation: The ==
operator compares the references of objects, whereas the equals()
method is typically overridden to compare the content of objects.
34. What is the purpose of the StringBuffer
class in Java?
a. To create immutable strings.
b. To create mutable strings with thread safety.
c. To represent a sequence of characters that cannot be modified.
d. To perform low-level input/output operations.
Correct Answer: b. To create mutable strings with thread safety.
Explanation: StringBuffer
in Java is used to create mutable strings, and it provides thread-safe operations.
35. What is the purpose of the ClassNotFoundException
in Java?
a. To handle exceptions when a class is not found at compile-time.
b. To handle exceptions when a class is not found at runtime.
c. To indicate that a class is not defined within a package.
d. To specify that a class is not accessible due to its access modifiers.
Correct Answer: b. To handle exceptions when a class is not found at runtime.
Explanation: ClassNotFoundException
in Java is thrown when an application tries to load a class dynamically at runtime, and the class is not found.
36. What is the purpose of the trim()
method in the String
class in Java?
a. To remove all whitespace characters from a string.
b. To truncate a string to a specified length.
c. To extract a substring from a string.
d. To remove leading and trailing whitespace from a string.
Correct Answer: d. To remove leading and trailing whitespace from a string.
Explanation: The trim()
method in Java is used to remove leading and trailing whitespace from a string.
37. How does the HashSet
class in Java handle duplicate elements?
a. It allows duplicate elements and retains all of them.
b. It does not allow duplicate elements and removes them automatically.
c. It throws an exception when duplicate elements are added.
d. It only retains the first occurrence of a duplicate element.
Correct Answer: b. It does not allow duplicate elements and removes them automatically.
Explanation: HashSet
in Java does not allow duplicate elements; if duplicates are added, they are automatically removed.
38. What is the purpose of the notify()
method in Java?
a. To wake up all waiting threads.
b. To release the current thread’s lock.
c. To notify the operating system about a thread’s status.
d. To wake up one of the waiting threads.
Correct Answer: d. To wake up one of the waiting threads.
Explanation: The notify()
method in Java is used to wake up one of the threads that are currently waiting on the object.
Explanation: The notify()
method in Java is used to wake up one of the threads that are currently waiting on the object.
46. What is the purpose of the super
keyword in Java?
a. To invoke the superclass method.
b. To access the superclass variable.
c. To refer to the current object.
d. To create a new object.
Correct Answer: a. To invoke the superclass method.
Explanation: The super
keyword in Java is used to invoke the method of the superclass.
47. What is the role of the finalize()
method in Java?
a. To force garbage collection for the object.
b. To indicate that an object is ready for destruction.
c. To release system resources before an object is reclaimed by the garbage collector.
d. To prevent an object from being collected by the garbage collector.
Correct Answer: c. To release system resources before an object is reclaimed by the garbage collector.
Explanation: The finalize()
method in Java is called by the garbage collector before it reclaims the memory occupied by an object, allowing the object to release system resources.
48. What is the purpose of the break
statement in Java?
a. To terminate the program.
b. To skip the current iteration and move to the next one.
c. To execute the code in the loop indefinitely.
d. To jump to a specific label within the loop.
Correct Answer: b. To skip the current iteration and move to the next one.
Explanation: The break
statement in Java is used to terminate the loop prematurely and move to the next iteration.
49. How does the StringBuilder
class differ from the String
class in Java?
a. StringBuilder
is immutable, while String
is mutable.
b. StringBuilder
is synchronized, while String
is not.
c. StringBuilder
is more memory-efficient for concatenating multiple strings.
d. StringBuilder
does not have a toString()
method.
Correct Answer: c. StringBuilder
is more memory-efficient for concatenating multiple strings.
Explanation: StringBuilder
in Java is mutable and is more memory-efficient than String
when performing multiple concatenations.
50. What is the purpose of the try-catch
block in Java?
a. To define a critical section of code.
b. To handle exceptions and provide a fallback mechanism.
c. To create a loop that continues until a condition is met.
d. To execute a block of code repeatedly.
Correct Answer: b. To handle exceptions and provide a fallback mechanism.
Explanation: The try-catch
block in Java is used to handle exceptions by providing alternative code to execute when an exception occurs.
51. What is the role of the this
keyword in Java?
a. To create a new object.
b. To refer to the current object.
c. To invoke the superclass method.
d. To access the superclass variable.
Correct Answer: b. To refer to the current object.
Explanation: The this
keyword in Java is used to refer to the current instance of the class.
52. How can you achieve encapsulation in Java?
a. By using the final
keyword.
b. By declaring all variables as public
.
c. By providing public getter and setter methods for private variables.
d. By using the static
keyword.
Correct Answer: c. By providing public getter and setter methods for private variables.
Explanation: Encapsulation in Java involves hiding the internal details of an object and restricting access to its state. This is often achieved by declaring variables as private and providing public getter and setter methods.
53. What is the purpose of the default
keyword in a Java interface?
a. To define a default implementation for a method in an interface.
b. To specify a default value for a variable in an interface.
c. To indicate that a method is optional and can be overridden by implementing classes.
d. To set a default visibility for the interface.
Correct Answer: a. To define a default implementation for a method in an interface.
Explanation: The default
keyword in Java interfaces is used to provide a default implementation for a method, allowing implementing classes to use it or override it.
54. How does the compareTo()
method in the Comparable
interface relate to sorting in Java?
a. It is used to reverse the natural order of elements.
b. It is called when a new element is added to a collection.
c. It defines the natural order of elements for sorting purposes.
d. It is used for comparing floating-point numbers.
Correct Answer: c. It defines the natural order of elements for sorting purposes.
Explanation: The compareTo()
method in the Comparable
interface is used to define the natural ordering of elements, allowing them to be sorted.
55. What is the purpose of the instanceof
operator in Java?
a. To check if an object is of a particular type at runtime.
b. To convert one type of object to another.
c. To create a new instance of a class.
d. To compare two objects for equality.
Correct Answer: a. To check if an object is of a particular type at runtime.
Explanation: The instanceof
operator in Java is used to test whether an object is an instance of a particular class or interface at runtime.
56. In Java, what is the purpose of the volatile
keyword when applied to a variable?
a. It ensures that the variable cannot be modified.
b. It guarantees atomicity for operations on the variable.
c. It prevents the variable from being cached by threads.
d. It allows the variable to be modified by multiple threads.
Correct Answer: d. It allows the variable to be modified by multiple threads.
Explanation: The volatile
keyword in Java is used to indicate that a variable’s value may be changed by multiple threads.
57. What is the purpose of the assert
statement in Java?
a. To check for null values in objects.
b. To handle exceptions in a concise manner.
c. To test code during development and provide a way to catch logical errors.
d. To define assertions for method arguments.
Correct Answer: c. To test code during development and provide a way to catch logical errors.
Explanation: The assert
statement in Java is used for testing code during development and catching logical errors.
58. How does the Enum
class in Java differ from a regular class?
a. Enums cannot have constructors.
b. Enums cannot have methods.
c. Enums cannot implement interfaces.
d. Enums have a fixed set of predefined instances.
Correct Answer: d. Enums have a fixed set of predefined instances.
Explanation: Enums in Java are a special type of class with a fixed set of predefined instances, representing a set of named values.
59. What is the purpose of the Runnable
interface in Java?
a. To define a class that can be serialized.
b. To create a thread by extending the Thread
class.
c. To define a task that can be executed by a thread.
d. To implement a single abstract method.
Correct Answer: c. To define a task that can be executed by a thread.
Explanation: The Runnable
interface in Java is used to define a task that can be executed by a thread.
60. What is the purpose of the NaN
(Not a Number) value in Java?
a. To represent infinity.
b. To indicate a missing value in numeric computations.
c. To signal an error in arithmetic operations.
d. To terminate a loop.
Correct Answer: b. To indicate a missing value in numeric computations.
Explanation: In Java, NaN
is used to represent a result that is undefined or unrepresentable in floating-point arithmetic.
61. How is the LinkedList
class different from the ArrayList
class in Java?
a. LinkedList
is more memory-efficient than ArrayList
.
b. ArrayList
allows faster random access to elements than LinkedList
.
c. LinkedList
allows elements to be easily inserted or removed in the middle of the list.
d. ArrayList
is a legacy class, while LinkedList
is modern.
Correct Answer: c. LinkedList
allows elements to be easily inserted or removed in the middle of the list.
Explanation: LinkedList
in Java allows elements to be easily inserted or removed in the middle of the list, while ArrayList
is better suited for random access.
62. What is the purpose of the Math.pow()
method in Java?
a. To calculate the square root of a number.
b. To raise a number to a specified power.
c. To calculate the logarithm of a number.
d. To round a floating-point number to the nearest integer.
Correct Answer: b. To raise a number to a specified power.
Explanation: The Math.pow()
method in Java is used to raise a number to the power of another number.
63. What is the significance of the super
keyword in a constructor in Java?
a. To invoke the constructor of the superclass.
b. To instantiate the subclass.
c. To reference the current object.
d. To create a new object.
Correct Answer: a. To invoke the constructor of the superclass.
Explanation: The super()
keyword in a constructor is used to invoke the constructor of the superclass from the constructor of the subclass.
64. How does the continue
statement differ from the break
statement in Java?
a. continue
is used to terminate a loop, while break
skips the current iteration.
b. break
is used to terminate a loop, while continue
skips the current iteration.
c. Both break
and continue
are used to terminate a loop.
d. Both break
and continue
are used to skip the current iteration.
Correct Answer: b. break
is used to terminate a loop, while continue
skips the current iteration.
Explanation: The break
statement is used to terminate a loop, while the continue
statement skips the current iteration and moves to the next one.
65. What is the purpose of the String.format()
method in Java?
a. To concatenate two strings.
b. To convert a string to uppercase.
c. To format a string using a specified format string and arguments.
d. To extract a substring from a string.
Correct Answer: c. To format a string using a specified format string and arguments.
Explanation: The String.format()
method in Java is used to format a string using a specified format string and arguments.
66. How can you create an immutable class in Java?
a. By making all methods final.
b. By making all methods static.
c. By declaring all variables as final and providing only getter methods.
d. By using the immutable
keyword.
Correct Answer: c. By declaring all variables as final and providing only getter methods.
Explanation: An immutable class in Java is created by declaring all variables as final and providing only getter methods.
Explanation: An immutable class in Java is created by declaring all variables as final and providing only getter methods.
73. How does the interface
keyword differ from the abstract
keyword in Java?
a. interface
is used to define abstract classes, while abstract
is used for interfaces.
b. interface
cannot have variables, while abstract
can.
c. interface
is used to declare a contract for classes to implement, while abstract
is used for creating abstract classes.
d. interface
can have constructors, while abstract
cannot.
Correct Answer: c. interface
is used to declare a contract for classes to implement, while abstract
is used for creating abstract classes.
Explanation: In Java, interface
is used to declare a contract for classes to implement, while abstract
is used for creating abstract classes with abstract methods.
74. What is the purpose of the try-with-resources
statement in Java?
a. To catch and handle exceptions in a concise manner.
b. To ensure that resources are closed after being used, automatically.
c. To create a try-catch block for critical sections of code.
d. To specify the scope of a try block.
Correct Answer: b. To ensure that resources are closed after being used, automatically.
Explanation: The try-with-resources
statement in Java is used for automatic resource management, ensuring that resources like files or sockets are closed after the try block is executed.
75. How can you prevent a class from being subclassed in Java?
a. By using the final
keyword on the class.
b. By using the abstract
keyword on the class.
c. By using the static
keyword on the class.
d. By using the sealed
keyword on the class.
Correct Answer: a. By using the final
keyword on the class.
Explanation: The final
keyword in Java is used to prevent a class from being subclassed.
76. What is the purpose of the break
statement within a loop in Java?
a. To exit the entire program.
b. To terminate the current iteration of the loop.
c. To exit the loop and resume normal program flow.
d. To skip the next iteration of the loop.
Correct Answer: c. To exit the loop and resume normal program flow.
Explanation: The break
statement within a loop in Java is used to exit the loop and resume normal program flow.
77. How does the this
keyword differ from the super
keyword in Java?
a. this
is used to invoke the superclass method, while super
is used to refer to the current object.
b. this
refers to the current object, while super
is used to refer to the superclass variable.
c. this
is used to create a new object, while super
is used to instantiate the subclass.
d. this
is used to access the superclass variable, while super
is used to create a new object.
Correct Answer: b. this
refers to the current object, while super
is used to refer to the superclass variable.
Explanation: In Java, this
refers to the current object, while super
is used to refer to the superclass variable.
78. What is the purpose of the EnumSet
class in Java?
a. To create a set of enum constants.
b. To represent a collection of elements with no duplicates.
c. To create a synchronized set of elements.
d. To create an ordered set of elements.
Correct Answer: a. To create a set of enum constants.
Explanation: The EnumSet
class in Java is used to create a set of enum constants.
79. What is the purpose of the try-catch
statement in Java?
a. To handle exceptions and provide a fallback mechanism.
b. To specify the scope of a try block.
c. To create a loop that continues until a condition is met.
d. To define a critical section of code.
Correct Answer: a. To handle exceptions and provide a fallback mechanism.
Explanation: The try-catch
statement in Java is used to handle exceptions by providing alternative code to execute when an exception occurs.
80. How does the instanceof
operator differ from type casting in Java?
a. instanceof
is used for primitive types, while type casting is used for objects.
b. instanceof
is used to check the type of an object at runtime, while type casting is used to convert between primitive types.
c. instanceof
is used to check the type of an object at compile-time, while type casting is used at runtime.
d. instanceof
and type casting are interchangeable; there is no difference.
Correct Answer: c. instanceof
is used to check the type of an object at runtime, while type casting is used at runtime.
Explanation: The instanceof
operator in Java is used to check the type of an object at runtime, while type casting is used for converting types at runtime.
81. How can you achieve multithreading in Java?
a. By using the synchronized
keyword.
b. By extending the Thread
class.
c. By implementing the Runnable
interface.
d. All of the above.
Correct Answer: d. All of the above.
Explanation: Multithreading in Java can be achieved by using the synchronized
keyword, extending the Thread
class, or implementing the Runnable
interface.
82. What is the purpose of the finalize()
method in Java?
a. To force garbage collection for the object.
b. To indicate that an object is ready for destruction.
c. To release system resources before an object is reclaimed by the garbage collector.
d. To prevent an object from being collected by the garbage collector.
Correct Answer: c. To release system resources before an object is reclaimed by the garbage collector.
Explanation: The finalize()
method in Java is called by the garbage collector before it reclaims the memory occupied by an object, allowing the object to release system resources.
83. What is the purpose of the transient
keyword in Java?
a. To indicate that a variable should not be serialized.
b. To specify that a variable should be initialized with a default value.
c. To make a variable accessible across different classes.
d. To declare a variable constant.
Correct Answer: a. To indicate that a variable should not be serialized.
Explanation: The transient
keyword in Java is used to indicate that a variable should not be included in the object’s serialized form.
84. How is method overloading different from method overriding in Java?
a. Overloaded methods have the same method signature, while overridden methods have different signatures.
b. Overloaded methods have different method names, while overridden methods have the same name.
c. Overloaded methods are used in inheritance, while overridden methods are not.
d. Overloaded methods are defined in the superclass, while overridden methods are defined in the subclass.
Correct Answer: b. Overloaded methods have different method names, while overridden methods have the same name.
Explanation: Method overloading involves having multiple methods with the same name but different parameters. Method overriding occurs when a subclass provides a specific implementation for a method defined in its superclass.
85. What is the purpose of the static
keyword in Java?
a. To make a variable or method applicable to the class rather than an instance.
b. To dynamically change the type of a variable.
c. To allow variables to be modified at runtime.
d. To enforce encapsulation.
Correct Answer: a. To make a variable or method applicable to the class rather than an instance.
Explanation: The static
keyword in Java is used to create variables and methods that belong to the class, not to the instance of the class.
93. What is the purpose of the System.arraycopy()
method in Java?
a. To copy the entire content of one array to another.
b. To copy a specified range of elements from one array to another.
c. To compare two arrays for equality.
d. To create a new array with a specified size.
Correct Answer: b. To copy a specified range of elements from one array to another.
Explanation: The System.arraycopy()
method in Java is used to copy a specified range of elements from one array to another.
94. How does the Random
class differ from the Math.random()
method in Java for generating random numbers?
a. Random
class is used for generating pseudo-random numbers, while Math.random()
uses true randomness.
b. Random
class provides more control and flexibility for generating random numbers.
c. Math.random()
is more efficient for generating random numbers.
d. Random
class is used for generating truly random numbers, while Math.random()
uses pseudo-randomness.
Correct Answer: a. Random
class is used for generating pseudo-random numbers, while Math.random()
uses true randomness.
Explanation: The Random
class in Java is used for generating pseudo-random numbers, while Math.random()
uses true randomness.
95. What is the purpose of the assert
statement in Java?
a. To check for null values in objects.
b. To handle exceptions in a concise manner.
c. To test code during development and provide a way to catch logical errors.
d. To define assertions for method arguments.
Correct Answer: c. To test code during development and provide a way to catch logical errors.
Explanation: The assert
statement in Java is used for testing code during development and catching logical errors.
96. How does the StringBuilder
class differ from the StringBuffer
class in Java?
a. StringBuilder
is synchronized, while StringBuffer
is not.
b. StringBuilder
is more memory-efficient for concatenating multiple strings.
c. StringBuilder
has a toString()
method, while StringBuffer
does not.
d. StringBuilder
is a legacy class, while StringBuffer
is modern.
Correct Answer: a. StringBuilder
is more memory-efficient for concatenating multiple strings.
Explanation: StringBuilder
in Java is not synchronized, making it more memory-efficient than StringBuffer
when performing multiple string concatenations.
97. What is the purpose of the instanceof
operator in Java?
a. To check if an object is of a particular type at runtime.
b. To convert one type of object to another.
c. To create a new instance of a class.
d. To compare two objects for equality.
Correct Answer: a. To check if an object is of a particular type at runtime.
Explanation: The instanceof
operator in Java is used to test whether an object is an instance of a particular class or interface at runtime.
98. How does the Enum
class in Java differ from an enumeration created using the enum
keyword?
a. Enum
class is used for creating enum constants, while the enum
keyword is used for creating classes.
b. Enum
class allows additional methods and fields, while the enum
keyword does not.
c. Enum
class is a legacy class, while the enum
keyword is modern.
d. Enum
class allows automatic generation of toString()
method, while the enum
keyword does not.
Correct Answer: b. Enum
class allows additional methods and fields, while the enum
keyword does not.
Explanation: The Enum
class in Java allows additional methods and fields, providing more flexibility compared to the basic enumeration created using the enum
keyword.
99. What is the purpose of the super()
constructor call in Java?
a. To create a new instance of the subclass.
b. To invoke the constructor of the superclass.
c. To instantiate the subclass.
d. To access the superclass variable.
Correct Answer: b. To invoke the constructor of the superclass.
Explanation: The super()
constructor call in Java is used to invoke the constructor of the superclass from the constructor of the subclass.
100. How can you achieve method overriding in Java?
a. By declaring a method as final
.
b. By using the static
keyword on the method.
c. By providing a specific implementation for a method in the subclass.
d. By using the abstract
keyword on the method.
Correct Answer: c. By providing a specific implementation for a method in the subclass.
Explanation: Method overriding in Java involves providing a specific implementation for a method in the subclass that is already defined in its superclass.
101. How does the try-with-resources
statement in Java improve resource management compared to traditional try-catch-finally?
a. It automatically releases system resources without the need for a finally
block.
b. It allows for better exception handling within the try
block.
c. It provides more control over resource management.
d. It is used for exception handling and not for resource management.
Correct Answer: a. It automatically releases system resources without the need for a finally
block.
Explanation: The try-with-resources
statement in Java automatically closes resources such as files or sockets when the try block completes, eliminating the need for a finally
block.
102. What is the purpose of the super
keyword in Java when used with variables?
a. To access the superclass variable.
b. To create a new variable.
c. To reference the current object.
d. To invoke the superclass method.
Correct Answer: a. To access the superclass variable.
Explanation: When used with variables, the super
keyword in Java is used to access the variable of the superclass.
103. How can you achieve encapsulation in Java?
a. By declaring all variables as public
.
b. By using the static
keyword on methods.
c. By declaring variables as private
and providing public getter and setter methods.
d. By using the final
keyword on variables.
Correct Answer: c. By declaring variables as private
and providing public getter and setter methods.
Explanation: Encapsulation in Java involves declaring variables as private
and providing public getter and setter methods to control access to the variables.
104. What is the purpose of the break
statement within a switch statement in Java?
a. To terminate the entire program.
b. To exit the switch statement and continue with the next iteration of the loop.
c. To terminate the loop containing the switch statement.
d. To exit the switch statement and resume normal program flow.
Correct Answer: d. To exit the switch statement and resume normal program flow.
Explanation: The break
statement within a switch statement in Java is used to exit the switch statement and resume normal program flow.
105. How does the equals()
method in Java differ from the ==
operator for comparing objects?
a. equals()
is used for primitive types, while ==
is used for objects.
b. equals()
compares the content of objects, while ==
checks if two references point to the same object.
c. equals()
is used for comparing integers, while ==
is used for floating-point numbers.
d. equals()
is used for reference types, while ==
is used for value types.
Correct Answer: b. equals()
compares the content of objects, while ==
checks if two references point to the same object.
Explanation: The equals()
method in Java is typically overridden to compare the content of objects, while the ==
operator checks if two references point to the same object.
106. What is the purpose of the static
keyword when applied to a variable in Java?
a. To indicate that the variable can be modified at runtime.
b. To create an instance variable.
c. To make the variable applicable to the class rather than an instance.
d. To enforce encapsulation.
Correct Answer: c. To make the variable applicable to the class rather than an instance.
Explanation: The static
keyword when applied to a variable in Java is used to make the variable applicable to the class rather than an instance.
107. How does the finally
block differ from the catch
block in a try-catch-finally statement in Java?
a. The finally
block is optional, while the catch
block is mandatory.
b. The catch
block is used for cleanup code, while the finally
block is used for exception handling.
c. The finally
block always executes, regardless of whether an exception is caught or not.
d. The catch
block always executes, regardless of whether an exception is caught or not.
Correct Answer: c. The finally
block always executes, regardless of whether an exception is caught or not.
Explanation: The finally
block in a try-catch-finally statement in Java always executes, regardless of whether an exception is caught or not.
108. How can you prevent a method from being overridden in a subclass in Java?
a. By declaring the method as final
.
b. By declaring the method as static
.
c. By declaring the method as abstract
.
d. By using the super
keyword.
Correct Answer: a. By declaring the method as final
.
Explanation: To prevent a method from being overridden in a subclass in Java, you can declare the method as final
.
117. How does the continue
statement differ from the return
statement in Java?
a. continue
is used to skip the rest of the code in a loop and move to the next iteration, while return
exits the entire method.
b. continue
exits the entire method, while return
skips the rest of the code in a loop and moves to the next iteration.
c. Both continue
and return
are used interchangeably.
d. continue
and return
serve the same purpose and can be used interchangeably.
Correct Answer: a. continue
is used to skip the rest of the code in a loop and move to the next iteration, while return
exits the entire method.
Explanation: The continue
statement in Java is used to skip the rest of the code in a loop and move to the next iteration, while the return
statement exits the entire method.
118. How can you handle concurrency issues in Java?
a. By using the synchronized
keyword.
b. By using the volatile
keyword.
c. By using locks from the java.util.concurrent
package.
d. All of the above.
Correct Answer: d. All of the above.
Explanation: Concurrency issues in Java can be handled by using the synchronized
keyword, the volatile
keyword, and locks from the java.util.concurrent
package.
119. What is the purpose of the instanceof
operator when dealing with polymorphism in Java?
a. To cast an object to a specific type.
b. To check if an object is an instance of a specific class or interface.
c. To compare two objects for equality.
d. To check if an object is null.
Correct Answer: b. To check if an object is an instance of a specific class or interface.
Explanation: The instanceof
operator in Java is used to check if an object is an instance of a specific class or interface.
120. How does the super
keyword differ from the this
keyword in Java?
a. super
refers to the current object, while this
is used to invoke the superclass method.
b. super
is used to access the superclass variable, while this
refers to the current object.
c. super
is used to create a new object, while this
is used to invoke the superclass method.
d. super
refers to the current object, while this
is used to access the superclass variable.
Correct Answer: b. super
is used to access the superclass variable, while this
refers to the current object.
Explanation: In Java, super
is used to access the superclass variable, while this
refers to the current object.
121. What is the purpose of the try-catch
statement in Java?
a. To catch and handle exceptions in a concise manner.
b. To ensure that resources are closed after being used.
c. To create a try-catch block for critical sections of code.
d. To specify the scope of a try block.
Correct Answer: a. To catch and handle exceptions in a concise manner.
Explanation: The try-catch
statement in Java is used to catch and handle exceptions in a concise manner by providing alternative code to execute when an exception occurs.
122. How does the static
keyword differ from the final
keyword in Java when applied to variables?
a. static
is used to declare constants, while final
is used for variables that can be modified at runtime.
b. static
is used for class-level variables, while final
is used for instance variables.
c. static
is used to make variables applicable to the class rather than an instance, while final
indicates that a variable cannot be modified.
d. static
and final
are interchangeable; there is no difference.
Correct Answer: c. static
is used to make variables applicable to the class rather than an instance, while final
indicates that a variable cannot be modified.
Explanation: When applied to variables in Java, static
is used to make variables applicable to the class rather than an instance, while final
indicates that a variable cannot be modified.
123. What is the purpose of the do-while
loop in Java?
a. To create an infinite loop.
b. To execute a block of code repeatedly while a condition is true.
c. To execute a block of code at least once, regardless of the condition.
d. To iterate over the elements of a collection.
Correct Answer: c. To execute a block of code at least once, regardless of the condition.
Explanation: The do-while
loop in Java is used to execute a block of code at least once, regardless of whether the condition is true or false.
124. How does the EnumSet
class in Java differ from other collection classes like HashSet
?
a. EnumSet
is used exclusively for enum constants, while HashSet
can store any type of object.
b. EnumSet
is synchronized, while HashSet
is not.
c. EnumSet
allows for efficient representation of a set of enum constants, while HashSet
is a general-purpose set implementation.
d. EnumSet
is part of the legacy collection framework, while HashSet
is modern.
Correct Answer: c. EnumSet
allows for efficient representation of a set of enum constants, while HashSet
is a general-purpose set implementation.
Explanation: EnumSet
in Java is designed specifically for efficiently representing a set of enum constants, providing performance benefits over general-purpose set implementations like HashSet
.
125. What is the purpose of the try-with-resources
statement in Java, and how does it improve resource management?
a. To explicitly close resources after they are used, improving memory management.
b. To automatically release system resources, such as files or sockets, without the need for a finally
block.
c. To catch exceptions that may occur during resource management.
d. To create a try-catch block for handling multiple resources.
Correct Answer: b. To automatically release system resources, such as files or sockets, without the need for a finally
block.
Explanation: The try-with-resources
statement in Java is used to automatically release system resources, such as files or sockets, without the need for an explicit finally
block.
126. How does the finalize()
method in Java differ from the close()
method when dealing with resource cleanup?
a. finalize()
is called explicitly by the programmer, while close()
is called by the garbage collector.
b. finalize()
is used for releasing system resources, while close()
is used for memory deallocation.
c. finalize()
is called by the garbage collector before an object is reclaimed, while close()
is called explicitly to release resources.
d. finalize()
is part of the AutoCloseable
interface, while close()
is part of the Object
class.
Correct Answer: c. finalize()
is called by the garbage collector before an object is reclaimed, while close()
is called explicitly to release resources.
Explanation: The finalize()
method in Java is called by the garbage collector before an object is reclaimed, typically used for resource cleanup. On the other hand, the close()
method is usually called explicitly by the programmer to release resources.
127. How does the break
statement behave when used within a loop in Java?
a. It terminates only the innermost loop.
b. It terminates all loops within which it is used.
c. It skips the current iteration of the loop.
d. It terminates the entire program.
Correct Answer: a. It terminates only the innermost loop.
Explanation: The break
statement in Java, when used within a loop, terminates only the innermost loop in which it is used.
128. How does the continue
statement differ from the break
statement in Java when used within a loop?
a. continue
terminates the entire loop, while break
skips the current iteration.
b. continue
skips the current iteration of the loop, while break
terminates the entire loop.
c. continue
and break
are interchangeable; there is no difference.
d. continue
and break
both terminate the entire program.
Correct Answer: b. continue
skips the current iteration of the loop, while break
terminates the entire loop.
Explanation: The continue
statement in Java skips the current iteration of the loop and continues with the next iteration, while the break
statement terminates the entire loop.
129. How does the Enum
class in Java differ from an enumeration created using the enum
keyword?
a. Enum
class is used for creating enum constants, while the enum
keyword is used for creating classes.
b. Enum
class allows additional methods and fields, while the enum
keyword does not.
c. Enum
class allows automatic generation of toString()
method, while the enum
keyword does not.
d. Enum
class is a legacy class, while the enum
keyword is modern.
Correct Answer: b. Enum
class allows additional methods and fields, while the enum
keyword does not.
Explanation: The Enum
class in Java allows additional methods and fields, providing more flexibility compared to the basic enumeration created using the enum
keyword.
130. How can you prevent a method from being overridden in a subclass in Java?
a. By declaring the method as final
.
b. By declaring the method as static
.
c. By declaring the method as abstract
.
d. By using the super
keyword.
Correct Answer: a. By declaring the method as final
.
Explanation: To prevent a method from being overridden in a subclass in Java, you can declare the method as final
.
131. How does the equals()
method in Java differ from the hashCode()
method?
a. equals()
is used for comparing primitive types, while hashCode()
is used for objects.
b. equals()
compares the content of objects, while hashCode()
generates a unique identifier for an object.
c. equals()
is used for reference types, while hashCode()
is used for value types.
d. equals()
is used for checking object identity, while hashCode()
is used for content comparison.
Correct Answer: b. equals()
compares the content of objects, while hashCode()
generates a unique identifier for an object.
Explanation: The equals()
method in Java is typically overridden to compare the content of objects, while the hashCode()
method generates a unique identifier for an object.
132. What is the purpose of the super()
constructor call in Java when used within a constructor?
a. To create a new instance of the subclass.
b. To invoke the constructor of the superclass.
c. To instantiate the subclass.
d. To access the superclass variable.
Correct Answer: b. To invoke the constructor of the superclass.
Explanation: The super()
constructor call in Java is used to invoke the constructor of the superclass from the constructor of the subclass.
133. How does the throw
statement differ from the throws
clause in Java?
a. throw
is used to declare that a method may throw an exception, while throws
is used to explicitly throw an exception.
b. throw
is used to rethrow an exception, while throws
is used to declare that a method may throw an exception.
c. throw
is used to catch and handle exceptions, while throws
is used to propagate exceptions.
d. throw
and throws
serve the same purpose and can be used interchangeably.
Correct Answer: b. throw
is used to rethrow an exception, while throws
is used to declare that a method may throw an exception.
Explanation: The throw
statement is used to throw an exception explicitly, while the throws
clause is used to declare that a method may throw an exception.
134. How does the static
keyword affect method overriding in Java?
a. It prevents the method from being overridden.
b. It enforces that the method must be overridden.
c. It allows the method to be overridden in a subclass.
d. It has no impact on method overriding.
Correct Answer: d. It has no impact on method overriding.
Explanation: The static
keyword in Java is not applicable to method overriding. It is used for class-level members and is not involved in the inheritance mechanism.
135. What is the purpose of the interface
keyword in Java?
a. To declare a new class.
b. To create an abstract class.
c. To define an interface with abstract methods.
d. To implement multiple inheritance.
Correct Answer: c. To define an interface with abstract methods.
Explanation: The interface
keyword in Java is used to define an interface, which is a collection of abstract methods and constants.
136. How does the super
keyword behave when used within a method in Java?
a. It refers to the current object.
b. It invokes the superclass method.
c. It creates a new instance of the superclass.
d. It initializes the superclass variable.
Correct Answer: b. It invokes the superclass method.
Explanation: When used within a method in Java, the super
keyword is used to invoke the method of the superclass.
137. How can you achieve multiple inheritance in Java?
a. By using the extends
keyword.
b. By using the implements
keyword.
c. By using the interface
keyword.
d. Java does not support multiple inheritance.
Correct Answer: d. Java does not support multiple inheritance.
Explanation: In Java, multiple inheritance (inheriting from more than one class) is not supported for classes. However, it is possible to achieve a form of multiple inheritance through interfaces.
138. How does the default
keyword in Java interfaces differ from the default
keyword in a switch statement?
a. default
in interfaces is used for specifying a default method implementation, while default
in a switch statement is used for handling unspecified cases.
b. default
in interfaces is used to declare default values for variables, while default
in a switch statement is used to provide a default case.
c. Both default
keywords serve the same purpose and can be used interchangeably.
d. default
in interfaces and default
in a switch statement are unrelated concepts.
Correct Answer: a. default
in interfaces is used for specifying a default method implementation, while default
in a switch statement is used for handling unspecified cases.
Explanation: In Java interfaces, the default
keyword is used to provide a default implementation for a method. In a switch statement, default
is used as a default case when no other case matches.
139. How does the instanceof
operator in Java behave when used with null?
a. It throws a NullPointerException
.
b. It always returns false
.
c. It always returns true
.
d. It compiles successfully but produces a warning.
Correct Answer: b. It always returns false
.
Explanation: When used with null, the instanceof
operator in Java always returns false
. It is a safe operation that does not throw a NullPointerException
.
140. What is the purpose of the synchronized
keyword in Java?
a. To prevent a method from being overridden.
b. To make a variable applicable to the class rather than an instance.
c. To control access to shared resources and prevent data corruption in multithreaded environments.
d. To declare a method as abstract.
Correct Answer: c. To control access to shared resources and prevent data corruption in multithreaded environments.
Explanation: The synchronized
keyword in Java is used to control access to shared resources and prevent data corruption in multithreaded environments.
141. What is the purpose of the super()
constructor call in Java when used within a constructor?
a. To create a new instance of the subclass.
b. To invoke the constructor of the superclass.
c. To instantiate the subclass.
d. To access the superclass variable.
Correct Answer: b. To invoke the constructor of the superclass.
Explanation: The super()
constructor call in Java is used to invoke the constructor of the superclass from the constructor of the subclass.
142. How does the throw
statement differ from the throws
clause in Java?
a. throw
is used to declare that a method may throw an exception, while throws
is used to explicitly throw an exception.
b. throw
is used to rethrow an exception, while throws
is used to declare that a method may throw an exception.
c. throw
is used to catch and handle exceptions, while throws
is used to propagate exceptions.
d. throw
and throws
serve the same purpose and can be used interchangeably.
Correct Answer: b. throw
is used to rethrow an exception, while throws
is used to declare that a method may throw an exception.
Explanation: The throw
statement is used to throw an exception explicitly, while the throws
clause is used to declare that a method may throw an exception.
143. How does the static
keyword affect method overriding in Java?
a. It prevents the method from being overridden.
b. It enforces that the method must be overridden.
c. It allows the method to be overridden in a subclass.
d. It has no impact on method overriding.
Correct Answer: d. It has no impact on method overriding.
Explanation: The static
keyword in Java is not applicable to method overriding. It is used for class-level members and is not involved in the inheritance mechanism.
144. What is the purpose of the interface
keyword in Java?
a. To declare a new class.
b. To create an abstract class.
c. To define an interface with abstract methods.
d. To implement multiple inheritance.
Correct Answer: c. To define an interface with abstract methods.
Explanation: The interface
keyword in Java is used to define an interface, which is a collection of abstract methods and constants.
145. How does the super
keyword behave when used within a method in Java?
a. It refers to the current object.
b. It invokes the superclass method.
c. It creates a new instance of the superclass.
d. It initializes the superclass variable.
Correct Answer: b. It invokes the superclass method.
Explanation: When used within a method in Java, the super
keyword is used to invoke the method of the superclass.
146. How can you achieve multiple inheritance in Java?
a. By using the extends
keyword.
b. By using the implements
keyword.
c. By using the interface
keyword.
d. Java does not support multiple inheritance.
Correct Answer: d. Java does not support multiple inheritance.
Explanation: In Java, multiple inheritance (inheriting from more than one class) is not supported for classes. However, it is possible to achieve a form of multiple inheritance through interfaces.
147. How does the default
keyword in Java interfaces differ from the default
keyword in a switch statement?
a. default
in interfaces is used for specifying a default method implementation, while default
in a switch statement is used for handling unspecified cases.
b. default
in interfaces is used to declare default values for variables, while default
in a switch statement is used to provide a default case.
c. Both default
keywords serve the same purpose and can be used interchangeably.
d. default
in interfaces and default
in a switch statement are unrelated concepts.
Correct Answer: a. default
in interfaces is used for specifying a default method implementation, while default
in a switch statement is used for handling unspecified cases.
Explanation: In Java interfaces, the default
keyword is used to provide a default implementation for a method. In a switch statement, default
is used as a default case when no other case matches.
148. How does the instanceof
operator in Java behave when used with null?
a. It throws a NullPointerException
.
b. It always returns false
.
c. It always returns true
.
d. It compiles successfully but produces a warning.
Correct Answer: b. It always returns false
.
Explanation: When used with null, the instanceof
operator in Java always returns false
. It is a safe operation that does not throw a NullPointerException
.
149. What is the purpose of the synchronized
keyword in Java?
a. To prevent a method from being overridden.
b. To make a variable applicable to the class rather than an instance.
c. To control access to shared resources and prevent data corruption in multithreaded environments.
d. To declare a method as abstract.
Correct Answer: c. To control access to shared resources and prevent data corruption in multithreaded environments.
Explanation: The synchronized
keyword in Java is used to control access to shared resources and prevent data corruption in multithreaded environments.
150. What is the purpose of the StringBuilder
class in Java, and how does it differ from String
in terms of mutability?
a. StringBuilder
is used for immutable string manipulation, while String
is mutable.
b. StringBuilder
is used for mutable string manipulation, while String
is immutable.
c. Both StringBuilder
and String
are immutable.
d. StringBuilder
and String
are unrelated classes.
Correct Answer: b. StringBuilder
is used for mutable string manipulation, while String
is immutable.
Explanation: The StringBuilder
class in Java is used for mutable string manipulation, allowing dynamic changes to the contents of a string. In contrast, the String
class is immutable, meaning its content cannot be changed after creation.
151. How does the continue
statement differ from the break
statement in Java when used within a loop?
a. continue
terminates the entire loop, while break
skips the current iteration.
b. continue
skips the current iteration of the loop, while break
terminates the entire loop.
c. continue
and break
are interchangeable; there is no difference.
d. continue
and break
both terminate the entire program.
Correct Answer: b. continue
skips the current iteration of the loop, while break
terminates the entire loop.
Explanation: The continue
statement in Java skips the current iteration of the loop and continues with the next iteration, while the break
statement terminates the entire loop.
152. What is the purpose of the try-with-resources
statement in Java, and how does it improve resource management?
a. To explicitly close resources after they are used, improving memory management.
b. To automatically release system resources, such as files or sockets, without the need for a finally
block.
c. To catch exceptions that may occur during resource management.
d. To create a try-catch block for handling multiple resources.
Correct Answer: b. To automatically release system resources, such as files or sockets, without the need for a finally
block.
Explanation: The try-with-resources
statement in Java is used to automatically release system resources, such as files or sockets, without the need for an explicit finally
block.
153. How does the default
keyword in a Java interface differ from the default
keyword in a switch statement?
a. default
in interfaces is used for specifying a default method implementation, while default
in a switch statement is used for handling unspecified cases.
b. default
in interfaces is used to declare default values for variables, while default
in a switch statement is used to provide a default case.
c. Both default
keywords serve the same purpose and can be used interchangeably.
d. default
in interfaces and default
in a switch statement are unrelated concepts.
Correct Answer: a. default
in interfaces is used for specifying a default method implementation, while default
in a switch statement is used for handling unspecified cases.
Explanation: In Java interfaces, the default
keyword is used to provide a default implementation for a method. In a switch statement, default
is used as a default case when no other case matches.
154. How does the finalize()
method in Java differ from the close()
method when dealing with resource cleanup?
a. finalize()
is called explicitly by the programmer, while close()
is called by the garbage collector.
b. finalize()
is used for releasing system resources, while close()
is used for memory deallocation.
c. finalize()
is called by the garbage collector before an object is reclaimed, while close()
is called explicitly to release resources.
d. finalize()
is part of the AutoCloseable
interface, while close()
is part of the Object
class.
Correct Answer: c. finalize()
is called by the garbage collector before an object is reclaimed, while close()
is called explicitly to release resources.
Explanation: The finalize()
method in Java is called by the garbage collector before an object is reclaimed, typically used for resource cleanup. On the other hand, the close()
method is usually called explicitly by the programmer to release resources.
155. How does the transient
keyword in Java affect the serialization process?
a. It excludes a variable from being serialized.
b. It indicates that a variable should be initialized with a default value.
c. It makes a variable serializable across different classes.
d. It prevents a variable from being accessed by other classes.
Correct Answer: a. It excludes a variable from being serialized.
Explanation: The transient
keyword in Java is used to exclude a variable from the serialization process, preventing it from being stored when the object is serialized.
156. How does the Enum
class in Java differ from an enumeration created using the enum
keyword?
a. Enum
class is used for creating enum constants, while the enum
keyword is used for creating classes.
b. Enum
class allows additional methods and fields, while the enum
keyword does not.
c. Enum
class allows automatic generation of toString()
method, while the enum
keyword does not.
d. Enum
class is a legacy class, while the enum
keyword is modern.
Correct Answer: b. Enum
class allows additional methods and fields, while the enum
keyword does not.
Explanation: The Enum
class in Java allows additional methods and fields, providing more flexibility compared to the basic enumeration created using the enum
keyword.
157. How does the equals()
method in Java differ from the hashCode()
method?
a. equals()
is used for comparing primitive types, while hashCode()
is used for objects.
b. equals()
compares the content of objects, while hashCode()
generates a unique identifier for an object.
c. equals()
is used for reference types, while hashCode()
is used for value types.
d. equals()
is used for checking object identity, while hashCode()
is used for content comparison.
Correct Answer: b. equals()
compares the content of objects, while hashCode()
generates a unique identifier for an object.
Explanation: The equals()
method in Java is typically overridden to compare the content of objects, while the hashCode()
method generates a unique identifier for an object.
158. How does the volatile
keyword in Java differ from the synchronized
keyword when dealing with multithreading?
a. volatile
ensures atomicity of a single read or write operation, while synchronized
ensures exclusive access to a block of code.
b. volatile
is used to make a variable applicable to the class rather than an instance, while synchronized
prevents a method from being overridden.
c. volatile
and synchronized
are interchangeable; there is no difference.
d. volatile
is used to create a new instance of the superclass, while synchronized
is used to control access to shared resources.
Correct Answer: a. volatile
ensures atomicity of a single read or write operation, while synchronized
ensures exclusive access to a block of code.
Explanation: The volatile
keyword in Java ensures the atomicity of a single read or write operation, while the synchronized
keyword ensures exclusive access to a block of code, preventing multiple threads from executing it simultaneously.
159. What is the purpose of the this
keyword in Java, and how does it differ from the super
keyword?
a. this
refers to the current object, while super
is used to invoke the superclass method.
b. this
is used to access the superclass variable, while super
refers to the current object.
c. this
is used to create a new object, while super
refers to the current object.
d. this
and super
are interchangeable; there is no difference.
Correct Answer: a. this
refers to the current object, while super
is used to invoke the superclass method.
Explanation: In Java, this
refers to the current object, allowing access to its members, while super
is used to invoke the method of the superclass.
160. How does the AutoCloseable
interface in Java contribute to resource management?
a. It provides a mechanism for automatically closing resources by implementing the close()
method.
b. It allows resources to be explicitly closed using the finalize()
method.
c. It is used for creating enum constants with automatic resource management.
d. It enforces the automatic release of system resources.
Correct Answer: a. It provides a mechanism for automatically closing resources by implementing the close()
method.
Explanation: The AutoCloseable
interface in Java provides a mechanism for automatically closing resources by implementing the close()
method. Objects that implement this interface can be used with the try-with-resources
statement for effective resource management.
161. How does the instanceof
operator in Java behave when used with an interface?
a. It throws a ClassCastException
if the object is not an instance of the interface.
b. It always returns true
if the object implements the interface.
c. It always returns false
if the object implements the interface.
d. It checks whether the object is an instance of the specified interface and returns a boolean result.
Correct Answer: d. It checks whether the object is an instance of the specified interface and returns a boolean result.
Explanation: The instanceof
operator in Java checks whether an object is an instance of a specified interface and returns a boolean result. It does not throw a ClassCastException
when used with interfaces.
162. How does the assert
statement in Java differ from other conditional statements like if
and switch
?
a. assert
is used for checking conditions at runtime, while if
and switch
are used for compile-time decisions.
b. assert
is used for handling exceptions, while if
and switch
are used for conditional branching.
c. assert
is used for testing purposes and can be disabled, while if
and switch
are always active.
d. assert
is used exclusively for integer comparisons, while if
and switch
work with any data type.
Correct Answer: c. assert
is used for testing purposes and can be disabled, while if
and switch
are always active.
Explanation: The assert
statement in Java is used for testing purposes and can be disabled at runtime using the -ea
(enable assertions) or -da
(disable assertions) option. In contrast, if
and switch
statements are always active.
163. How does the super
keyword differ from the this
keyword in Java when used within a constructor?
a. super
refers to the current object, while this
is used to invoke the superclass method.
b. super
is used to access the superclass variable, while this
refers to the current object.
c. super
is used to create a new object, while this
refers to the current object.
d. super
and this
are interchangeable; there is no difference.
Correct Answer: c. super
is used to create a new object, while this
refers to the current object.
Explanation: When used within a constructor in Java, super
is used to invoke the constructor of the superclass and create a new object, while this
refers to the current object being constructed.
164. How does the do-while
loop in Java differ from the while
loop?
a. do-while
is used for infinite loops, while while
is used for a fixed number of iterations.
b. do-while
guarantees that the loop body is executed at least once, while while
may skip the first iteration.
c. do-while
and while
are interchangeable; there is no difference.
d. do-while
is not part of the Java programming language.
Correct Answer: b. do-while
guarantees that the loop body is executed at least once, while while
may skip the first iteration.
Explanation: The do-while
loop in Java guarantees that the loop body is executed at least once because the condition is checked after the loop body. In contrast, the while
loop may skip the first iteration if the condition is initially false.
165. How does the final
keyword in Java differ from the finally
block?
a. final
is used for declaring a constant variable, while finally
is used for resource cleanup.
b. final
is a block that always executes, while finally
is used for marking the end of a class.
c. final
is used for preventing method overriding, while finally
is a block that always executes after a try-catch block.
d. final
and finally
are interchangeable; there is no difference.
Correct Answer: c. final
is used for preventing method overriding, while finally
is a block that always executes after a try-catch block.
Explanation: In Java, the final
keyword is used to prevent method overriding or to declare constants, while the finally
block is used to specify code that always executes after a try-catch block, regardless of whether an exception is thrown or not.
Explanation: In Java, the final
keyword is used to prevent method overriding or to declare constants, while the finally
block is used to specify code that always executes after a try-catch block, regardless of whether an exception is thrown or not.
174. How does the transient
keyword in Java affect the serialization process?
a. It excludes a variable from being serialized.
b. It indicates that a variable should be initialized with a default value.
c. It makes a variable serializable across different classes.
d. It prevents a variable from being accessed by other classes.
Correct Answer: a. It excludes a variable from being serialized.
Explanation: The transient
keyword in Java is used to exclude a variable from the serialization process, preventing it from being stored when the object is serialized.
175. How does the continue
statement differ from the break
statement in Java when used within a loop?
a. continue
terminates the entire loop, while break
skips the current iteration.
b. continue
skips the current iteration of the loop, while break
terminates the entire loop.
c. continue
and break
are interchangeable; there is no difference.
d. continue
and break
both terminate the entire program.
Correct Answer: b. continue
skips the current iteration of the loop, while break
terminates the entire loop.
Explanation: The continue
statement in Java skips the current iteration of the loop and continues with the next iteration, while the break
statement terminates the entire loop.
176. How does the default
keyword in a Java interface differ from the default
keyword in a switch statement?
a. default
in interfaces is used for specifying a default method implementation, while default
in a switch statement is used for handling unspecified cases.
b. default
in interfaces is used to declare default values for variables, while default
in a switch statement is used to provide a default case.
c. Both default
keywords serve the same purpose and can be used interchangeably.
d. default
in interfaces and default
in a switch statement are unrelated concepts.
Correct Answer: a. default
in interfaces is used for specifying a default method implementation, while default
in a switch statement is used for handling unspecified cases.
Explanation: In Java interfaces, the default
keyword is used to provide a default implementation for a method. In a switch statement, default
is used as a default case when no other case matches.
177. How does the AutoCloseable
interface in Java contribute to resource management?
a. It provides a mechanism for automatically closing resources by implementing the close()
method.
b. It allows resources to be explicitly closed using the finalize()
method.
c. It is used for creating enum constants with automatic resource management.
d. It enforces the automatic release of system resources.
Correct Answer: a. It provides a mechanism for automatically closing resources by implementing the close()
method.
Explanation: The AutoCloseable
interface in Java provides a mechanism for automatically closing resources by implementing the close()
method. Objects that implement this interface can be used with the try-with-resources
statement for effective resource management.
178. How does the instanceof
operator in Java behave when used with an interface?
a. It throws a ClassCastException
if the object is not an instance of the interface.
b. It always returns true
if the object implements the interface.
c. It always returns false
if the object implements the interface.
d. It checks whether the object is an instance of the specified interface and returns a boolean result.
Correct Answer: d. It checks whether the object is an instance of the specified interface and returns a boolean result.
Explanation: The instanceof
operator in Java checks whether an object is an instance of a specified interface and returns a boolean result. It does not throw a ClassCastException
when used with interfaces.
179. How does the assert
statement in Java differ from other conditional statements like if
and switch
?
a. assert
is used for checking conditions at runtime, while if
and switch
are used for compile-time decisions.
b. assert
is used for handling exceptions, while if
and switch
are used for conditional branching.
c. assert
is used for testing purposes and can be disabled, while if
and switch
are always active.
d. assert
is used exclusively for integer comparisons, while if
and switch
work with any data type.
Correct Answer: c. assert
is used for testing purposes and can be disabled, while if
and switch
are always active.
Explanation: The assert
statement in Java is used for testing purposes and can be disabled at runtime using the -ea
(enable assertions) or -da
(disable assertions) option. In contrast, if
and switch
statements are always active.
180. How does the finalize()
method in Java differ from the close()
method when dealing with resource cleanup?
a. finalize()
is called explicitly by the programmer, while close()
is called by the garbage collector.
b. finalize()
is used for releasing system resources, while close()
is used for memory deallocation.
c. finalize()
is called by the garbage collector before an object is reclaimed, while close()
is called explicitly to release resources.
d. finalize()
is part of the AutoCloseable
interface, while close()
is part of the Object
class.
Correct Answer: c. finalize()
is called by the garbage collector before an object is reclaimed, while close()
is called explicitly to release resources.
Explanation: The finalize()
method in Java is called by the garbage collector before an object is reclaimed, typically used for resource cleanup. On the other hand, the close()
method is usually called explicitly by the programmer to release resources.
181. How does the volatile
keyword in Java differ from the synchronized
keyword when dealing with multithreading?
a. volatile
ensures atomicity of a single read or write operation, while synchronized
ensures exclusive access to a block of code.
b. volatile
is used to make a variable applicable to the class rather than an instance, while synchronized
prevents a method from being overridden.
c. volatile
and synchronized
are interchangeable; there is no difference.
d. volatile
is used to create a new instance of the superclass, while synchronized
is used to control access to shared resources.
Correct Answer: a. volatile
ensures atomicity of a single read or write operation, while synchronized
ensures exclusive access to a block of code.
Explanation: The volatile
keyword in Java ensures the atomicity of a single read or write operation, while the synchronized
keyword ensures exclusive access to a block of code, preventing multiple threads from executing it simultaneously.
182. What is the purpose of the this
keyword in Java, and how does it differ from the super
keyword?
a. this
refers to the current object, while super
is used to invoke the superclass method.
b. this
is used to access the superclass variable, while super
refers to the current object.
c. this
is used to create a new object, while super
refers to the current object.
d. this
and super
are interchangeable; there is no difference.
Correct Answer: a. this
refers to the current object, while super
is used to invoke the superclass method.
Explanation: In Java, this
refers to the current object, allowing access to its members, while super
is used to invoke the method of the superclass.
183. How does the AutoCloseable
interface in Java contribute to resource management?
a. It provides a mechanism for automatically closing resources by implementing the close()
method.
b. It allows resources to be explicitly closed using the finalize()
method.
c. It is used for creating enum constants with automatic resource management.
d. It enforces the automatic release of system resources.
Correct Answer: a. It provides a mechanism for automatically closing resources by implementing the close()
method.
Explanation: The AutoCloseable
interface in Java provides a mechanism for automatically closing resources by implementing the close()
method. Objects that implement this interface can be used with the try-with-resources
statement for effective resource management.
184. How does the instanceof
operator in Java behave when used with an interface?
a. It throws a ClassCastException
if the object is not an instance of the interface.
b. It always returns true
if the object implements the interface.
c. It always returns false
if the object implements the interface.
d. It checks whether the object is an instance of the specified interface and returns a boolean result.
Correct Answer: d. It checks whether the object is an instance of the specified interface and returns a boolean result.
Explanation: The instanceof
operator in Java checks whether an object is an instance of a specified interface and returns a boolean result. It does not throw a ClassCastException
when used with interfaces.
185. How does the assert
statement in Java differ from other conditional statements like if
and switch
?
a. assert
is used for checking conditions at runtime, while if
and switch
are used for compile-time decisions.
b. assert
is used for handling exceptions, while if
and switch
are used for conditional branching.
c. assert
is used for testing purposes and can be disabled, while if
and switch
are always active.
d. assert
is used exclusively for integer comparisons, while if
and switch
work with any data type.
Correct Answer: c. assert
is used for testing purposes and can be disabled, while if
and switch
are always active.
Explanation: The assert
statement in Java is used for testing purposes and can be disabled at runtime using the -ea
(enable assertions) or -da
(disable assertions) option. In contrast, if
and switch
statements are always active.
186. How does the super
keyword differ from the this
keyword in Java when used within a constructor?
a. super
refers to the current object, while this
is used to invoke the superclass method.
b. super
is used to access the superclass variable, while this
refers to the current object.
c. super
is used to create a new object, while this
refers to the current object.
d. super
and this
are interchangeable; there is no difference.
Correct Answer: c. super
is used to create a new object, while this
refers to the current object.
Explanation: When used within a constructor in Java, super
is used to invoke the constructor of the superclass and create a new object, while this
refers to the current object being constructed.
187. How does the do-while
loop in Java differ from the while
loop?
a. do-while
is used for infinite loops, while while
is used for a fixed number of iterations.
b. do-while
guarantees that the loop body is executed at least once, while while
may skip the first iteration.
c. do-while
and while
are interchangeable; there is no difference.
d. do-while
is not part of the Java programming language.
Correct Answer: b. do-while
guarantees that the loop body is executed at least once, while while
may skip the first iteration.
Explanation: The do-while
loop in Java guarantees that the loop body is executed at least once because the condition is checked after the loop body. In contrast, the while
loop may skip the first iteration if the condition is initially false.
188. How does the final
keyword in Java differ from the finally
block?
a. final
is used for declaring a constant variable, while finally
is used for resource cleanup.
b. final
is a block that always executes, while finally
is used for marking the end of a class.
c. final
is used for preventing method overriding, while finally
is a block that always executes after a try-catch block.
d. final
and finally
are interchangeable; there is no difference.
Correct Answer: c. final
is used for preventing method overriding, while finally
is a block that always executes after a try-catch block.
Explanation: In Java, the final
keyword is used to prevent method overriding or to declare constants, while the finally
block is used to specify code that always executes after a try-catch block, regardless of whether an exception is thrown or not.
189. How does the throw
statement differ from the throws
clause in Java?
a. throw
is used to declare that a method may throw an exception, while throws
is used to explicitly throw an exception.
b. throw
is used to rethrow an exception, while throws
is used to declare that a method may throw an exception.
c. throw
is used to catch and handle exceptions, while throws
is used to propagate exceptions.
d. throw
and throws
serve the same purpose and can be used interchangeably.
Correct Answer: b. throw
is used to rethrow an exception, while throws
is used to declare that a method may throw an exception.
Explanation: The throw
statement in Java is used to throw an exception explicitly, while the throws
clause is used to declare that a method may throw an exception.
190. How does the static
keyword affect method overriding in Java?
a. It prevents the method from being overridden.
b. It enforces that the method must be overridden.
c. It allows the method to be overridden in a subclass.
d. It has no impact on method overriding.
Correct Answer: d. It has no impact on method overriding.
Explanation: The static
keyword in Java is not applicable to method overriding. It is used for class-level members and is not involved in the inheritance mechanism.
191. What is the purpose of the interface
keyword in Java?
a. To declare a new class.
b. To create an abstract class.
c. To define an interface with abstract methods.
d. To implement multiple inheritance.
Correct Answer: c. To define an interface with abstract methods.
Explanation: The interface
keyword in Java is used to define an interface, which is a collection of abstract methods and constants.
192. How does the super
keyword behave when used within a method in Java?
a. It refers to the current object.
b. It invokes the superclass method.
c. It creates a new instance of the superclass.
d. It initializes the superclass variable.
Correct Answer: b. It invokes the superclass method.
Explanation: When used within a method in Java, the super
keyword is used to invoke the method of the superclass.
193. How can you prevent a method from being overridden in a subclass in Java?
a. By declaring the method as final
.
b. By declaring the method as static
.
c. By declaring the method as abstract
.
d. By using the super
keyword.
Correct Answer: a. By declaring the method as final
.
Explanation: To prevent a method from being overridden in a subclass in Java, you can declare the method as final
.
194. How does the Enum
class in Java differ from an enumeration created using the enum
keyword?
a. Enum
class is used for creating enum constants, while the enum
keyword is used for creating classes.
b. Enum
class allows additional methods and fields, while the enum
keyword does not.
c. Enum
class allows automatic generation of toString()
method, while the enum
keyword does not.
d. Enum
class is a legacy class, while the enum
keyword is modern.
Correct Answer: b. Enum
class allows additional methods and fields, while the enum
keyword does not.
Explanation: The Enum
class in Java allows additional methods and fields, providing more flexibility compared to the basic enumeration created using the enum
keyword.
195. How can you handle checked exceptions in Java?
a. By using the throws
clause.
b. By using the try-catch
block.
c. By using the finally
block.
d. By using the throw
statement.
Correct Answer: b. By using the try-catch
block.
Explanation: Checked exceptions in Java must be either caught using a try-catch
block or declared in the method signature using the throws
clause.
196. How does the this
keyword in Java differ from the super
keyword when used within a constructor?
a. this
refers to the current object, while super
is used to invoke the superclass method.
b. this
is used to access the superclass variable, while super
refers to the current object.
c. this
is used to create a new object, while super
refers to the current object.
d. this
and super
are interchangeable; there is no difference.
Correct Answer: c. this
is used to create a new object, while super
refers to the current object.
Explanation: When used within a constructor in Java, this
is used to refer to the current object being constructed, while super
is used to invoke the constructor of the superclass and create a new object.
197. How does the transient
keyword in Java affect the serialization process?
a. It excludes a variable from being serialized.
b. It indicates that a variable should be initialized with a default value.
c. It makes a variable serializable across different classes.
d. It prevents a variable from being accessed by other classes.
Correct Answer: a. It excludes a variable from being serialized.
Explanation: The transient
keyword in Java is used to exclude a variable from the serialization process, preventing it from being stored when the object is serialized.
198. How does the continue
statement differ from the break
statement in Java when used within a loop?
a. continue
terminates the entire loop, while break
skips the current iteration.
b. continue
skips the current iteration of the loop, while break
terminates the entire loop.
c. continue
and break
are interchangeable; there is no difference.
d. continue
and break
both terminate the entire program.
Correct Answer: b. continue
skips the current iteration of the loop, while break
terminates the entire loop.
Explanation: The continue
statement in Java skips the current iteration of the loop and continues with the next iteration, while the break
statement terminates the entire loop.
199. How does the default
keyword in a Java interface differ from the default
keyword in a switch statement?
a. default
in interfaces is used for specifying a default method implementation, while default
in a switch statement is used for handling unspecified cases.
b. default
in interfaces is used to declare default values for variables, while default
in a switch statement is used to provide a default case.
c. Both default
keywords serve the same purpose and can be used interchangeably.
d. default
in interfaces and default
in a switch statement are unrelated concepts.
Correct Answer: a. default
in interfaces is used for specifying a default method implementation, while default
in a switch statement is used for handling unspecified cases.
Explanation: In Java interfaces, the default
keyword is used to provide a default implementation for a method. In a switch statement, default
is used as a default case when no other case matches.
200. How does the AutoCloseable
interface in Java contribute to resource management?
a. It provides a mechanism for automatically closing resources by implementing the close()
method.
b. It allows resources to be explicitly closed using the finalize()
method.
c. It is used for creating enum constants with automatic resource management.
d. It enforces the automatic release of system resources.
Correct Answer: a. It provides a mechanism for automatically closing resources by implementing the close()
method.
Explanation: The AutoCloseable
interface in Java provides a mechanism for automatically closing resources by implementing the close()
method. Objects that implement this interface can be used with the try-with-resources
statement for effective resource management.
201. How does the finalize()
method in Java differ from the close()
method when dealing with resource cleanup?
a. finalize()
is called explicitly by the programmer, while close()
is called by the garbage collector.
b. finalize()
is used for releasing system resources, while close()
is used for memory deallocation.
c. finalize()
is called by the garbage collector before an object is reclaimed, while close()
is called explicitly to release resources.
d. finalize()
is part of the AutoCloseable
interface, while close()
is part of the Object
class.
Correct Answer: c. finalize()
is called by the garbage collector before an object is reclaimed, while close()
is called explicitly to release resources.
Explanation: The finalize()
method in Java is called by the garbage collector before an object is reclaimed, typically used for resource cleanup. On the other hand, the close()
method is usually called explicitly by the programmer to release resources.
202. How does the volatile
keyword in Java differ from the synchronized
keyword when dealing with multithreading?
a. volatile
ensures atomicity of a single read or write operation, while synchronized
ensures exclusive access to a block of code.
b. volatile
is used to make a variable applicable to the class rather than an instance, while synchronized
prevents a method from being overridden.
c. volatile
and synchronized
are interchangeable; there is no difference.
d. volatile
is used to create a new instance of the superclass, while synchronized
is used to control access to shared resources.
Correct Answer: a. volatile
ensures atomicity of a single read or write operation, while synchronized
ensures exclusive access to a block of code.
Explanation: The volatile
keyword in Java ensures the atomicity of a single read or write operation, while the synchronized
keyword ensures exclusive access to a block of code, preventing multiple threads from executing it simultaneously.
203. What is the purpose of the this
keyword in Java, and how does it differ from the super
keyword?
a. this
refers to the current object, while super
is used to invoke the superclass method.
b. this
is used to access the superclass variable, while super
refers to the current object.
c. this
is used to create a new object, while super
refers to the current object.
d. this
and super
are interchangeable; there is no difference.
Correct Answer: a. this
refers to the current object, while super
is used to invoke the superclass method.
Explanation: In Java, this
refers to the current object, allowing access to its members, while super
is used to invoke the method of the superclass.
204. How does the AutoCloseable
interface in Java contribute to resource management?
a. It provides a mechanism for automatically closing resources by implementing the close()
method.
b. It allows resources to be explicitly closed using the finalize()
method.
c. It is used for creating enum constants with automatic resource management.
d. It enforces the automatic release of system resources.
Correct Answer: a. It provides a mechanism for automatically closing resources by implementing the close()
method.
Explanation: The AutoCloseable
interface in Java provides a mechanism for automatically closing resources by implementing the close()
method. Objects that implement this interface can be used with the try-with-resources
statement for effective resource management.
205. How does the instanceof
operator in Java behave when used with an interface?
a. It throws a ClassCastException
if the object is not an instance of the interface.
b. It always returns true
if the object implements the interface.
c. It always returns false
if the object implements the interface.
d. It checks whether the object is an instance of the specified interface and returns a boolean result.
Correct Answer: d. It checks whether the object is an instance of the specified interface and returns a boolean result.
Explanation: The instanceof
operator in Java checks whether an object is an instance of a specified interface and returns a boolean result. It does not throw a ClassCastException
when used with interfaces.
206. How does the assert
statement in Java differ from other conditional statements like if
and switch
?
a. assert
is used for checking conditions at runtime, while if
and switch
are used for compile-time decisions.
b. assert
is used for handling exceptions, while if
and switch
are used for conditional branching.
c. assert
is used for testing purposes and can be disabled, while if
and switch
are always active.
d. assert
is used exclusively for integer comparisons, while if
and switch
work with any data type.
Correct Answer: c. assert
is used for testing purposes and can be disabled, while if
and switch
are always active.
Explanation: The assert
statement in Java is used for testing purposes and can be disabled at runtime using the -ea
(enable assertions) or -da
(disable assertions) option. In contrast, if
and switch
statements are always active.
207. How does the super
keyword differ from the this
keyword in Java when used within a constructor?
a. super
refers to the current object, while this
is used to invoke the superclass method.
b. super
is used to access the superclass variable, while this
refers to the current object.
c. super
is used to create a new object, while this
refers to the current object.
d. super
and this
are interchangeable; there is no difference.
Correct Answer: c. super
is used to create a new object, while this
refers to the current object.
Explanation: When used within a constructor in Java, super
is used to invoke the constructor of the superclass and create a new object, while this
refers to the current object being constructed.
208. How does the do-while
loop in Java differ from the while
loop?
a. do-while
is used for infinite loops, while while
is used for a fixed number of iterations.
b. do-while
guarantees that the loop body is executed at least once, while while
may skip the first iteration.
c. do-while
and while
are interchangeable; there is no difference.
d. do-while
is not part of the Java programming language.
Correct Answer: b. do-while
guarantees that the loop body is executed at least once, while while
may skip the first iteration.
Explanation: The do-while
loop in Java guarantees that the loop body is executed at least once because the condition is checked after the loop body. In contrast, the while
loop may skip the first iteration if the condition is initially false.