Introduction: Good morning, students! Today, we will learn about creating tables in HTML. Tables are a fundamental part of web design used to display data in rows and columns, making it easy to understand and compare information. By the end of this lecture, you will know how to create, format, and style tables in HTML.
1. Basic Structure of an HTML Table:
An HTML table is created using the <table>
tag. Inside the <table>
tag, we use several other tags to define the structure of the table:
<tr>
: Table row<th>
: Table header cell<td>
: Table data cell
Let’s start with a simple example:
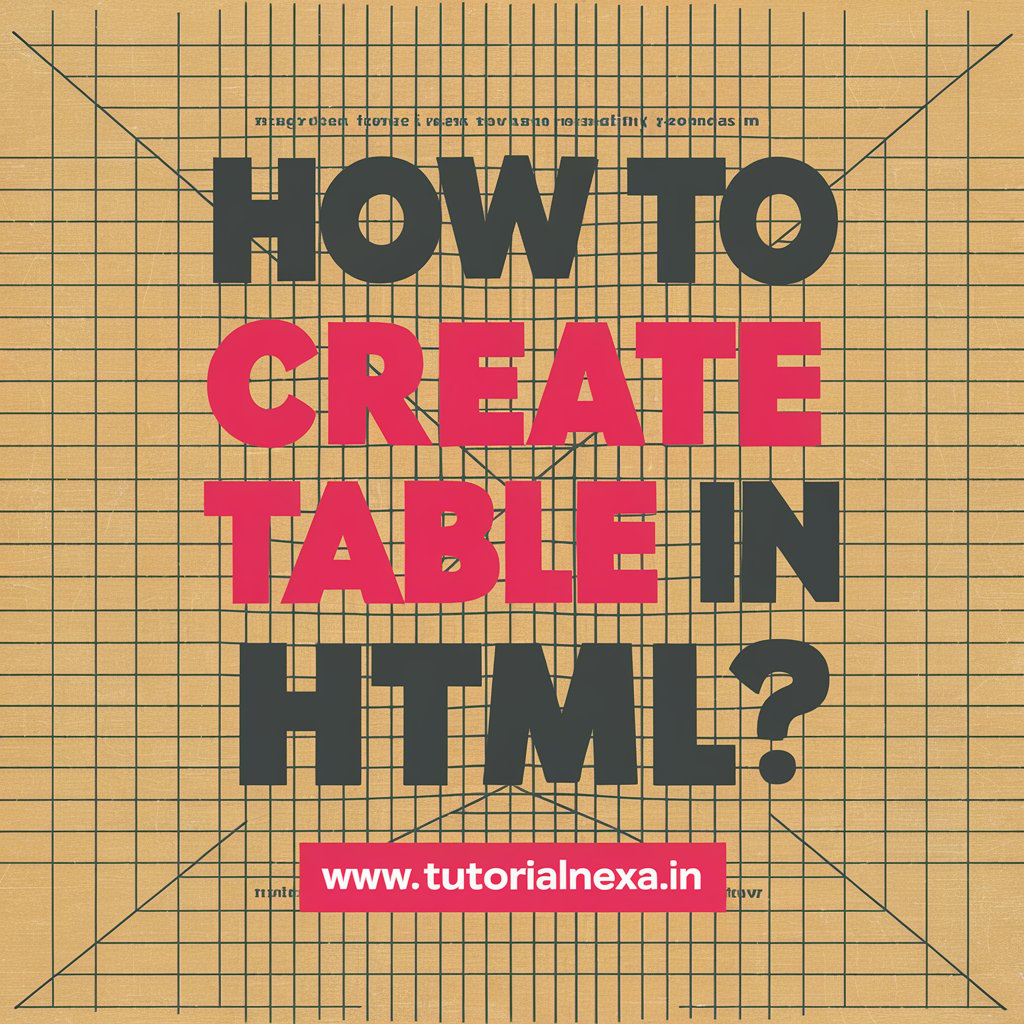
<!DOCTYPE html>
<html>
<head>
<title>HTML Table Example</title>
</head>
<body>
<table border="1">
<tr>
<th>Name</th>
<th>Age</th>
<th>City</th>
</tr>
<tr>
<td>John</td>
<td>25</td>
<td>New York</td>
</tr>
<tr>
<td>Jane</td>
<td>30</td>
<td>Los Angeles</td>
</tr>
</table>
</body>
</html>
Explanation:
- The
<table>
tag defines the start of the table. - The
border="1"
attribute adds a border to the table. - The
<tr>
tag creates a new row. - The
<th>
tag creates a header cell. By default, text in a<th>
element is bold and centered. - The
<td>
tag creates a data cell.
2. Adding Table Headers and Captions:
Headers are essential for understanding the content of each column. Captions can be added to tables using the <caption>
tag.
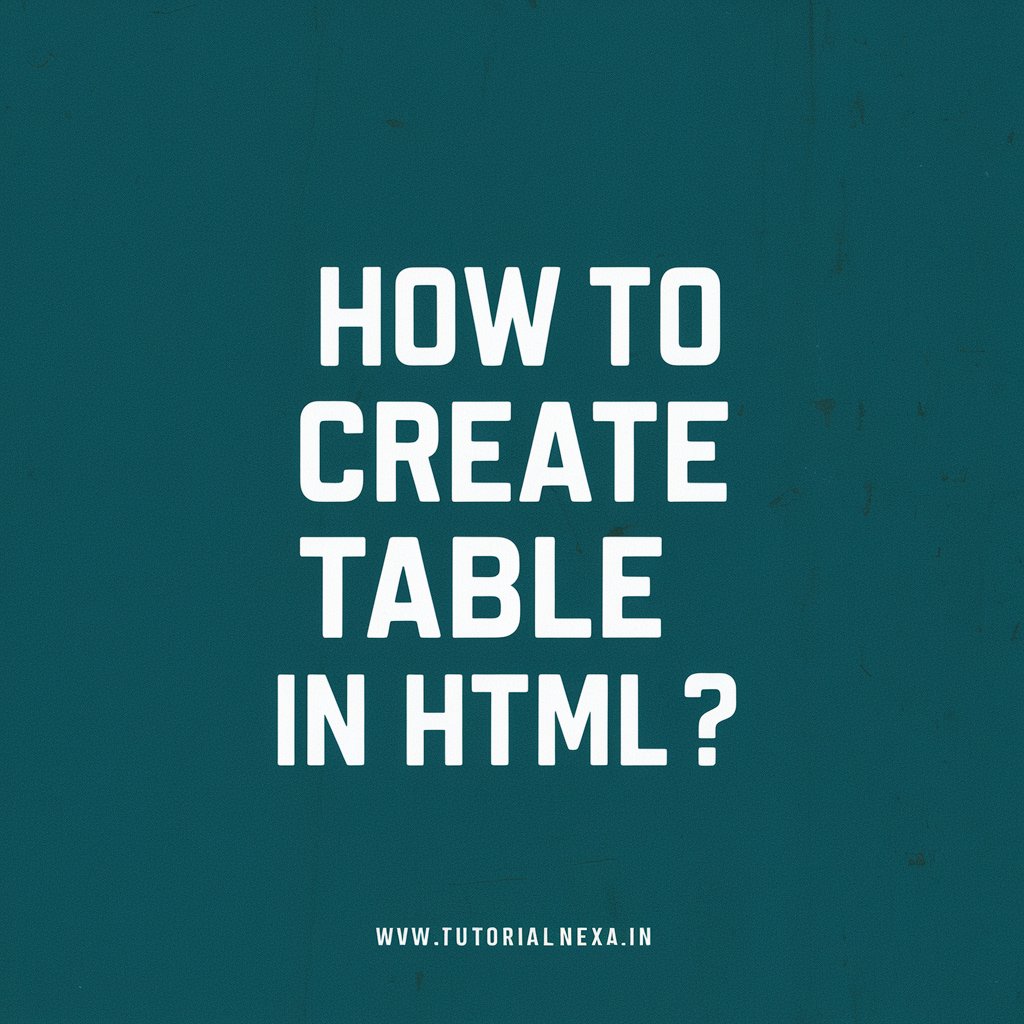
<!DOCTYPE html>
<html>
<head>
<title>HTML Table with Caption</title>
</head>
<body>
<table border="1">
<caption>Employee Information</caption>
<tr>
<th>Name</th>
<th>Age</th>
<th>City</th>
</tr>
<tr>
<td>John</td>
<td>25</td>
<td>New York</td>
</tr>
<tr>
<td>Jane</td>
<td>30</td>
<td>Los Angeles</td>
</tr>
</table>
</body>
</html>
explanation:
- The
<caption>
tag adds a title to the table, making it more descriptive.
3. Spanning Rows and Columns:
Sometimes, you may want a cell to span multiple columns or rows. This is achieved using the colspan
and rowspan
attributes.
<!DOCTYPE html>
<html>
<head>
<title>HTML Table with Span</title>
</head>
<body>
<table border="1">
<tr>
<th>Name</th>
<th colspan="2">Details</th>
</tr>
<tr>
<td>John</td>
<td>25</td>
<td>New York</td>
</tr>
<tr>
<td rowspan="2">Jane</td>
<td>30</td>
<td>Los Angeles</td>
</tr>
<tr>
<td>31</td>
<td>San Francisco</td>
</tr>
</table>
</body>
</html>
Explanation:
colspan="2"
makes the header cell span across two columns.rowspan="2"
makes the data cell span across two rows.
4. Styling Tables:
Tables can be styled using CSS to make them more visually appealing.
<!DOCTYPE html>
<html>
<head>
<title>Styled HTML Table</title>
<style>
table {
width: 50%;
border-collapse: collapse;
}
th, td {
padding: 8px;
text-align: left;
border-bottom: 1px solid #ddd;
}
th {
background-color: #f2f2f2;
}
tr:hover {
background-color: #f5f5f5;
}
</style>
</head>
<body>
<table>
<caption>Styled Employee Information</caption>
<tr>
<th>Name</th>
<th>Age</th>
<th>City</th>
</tr>
<tr>
<td>John</td>
<td>25</td>
<td>New York</td>
</tr>
<tr>
<td>Jane</td>
<td>30</td>
<td>Los Angeles</td>
</tr>
</table>
</body>
</html>
Styled HTML Table
Name | Age | City |
---|---|---|
John | 25 | New York |
Jane | 30 | Los Angeles |
Explanation:
- The
width
property sets the width of the table. border-collapse: collapse;
removes the space between table cells.padding
adds space inside each cell.border-bottom
adds a bottom border to each cell.background-color
sets the background color.tr:hover
changes the background color when hovering over a row.
5. Best Practices:
- Use
<thead>
,<tbody>
, and<tfoot>
for better structure and readability. - Always include a
<caption>
for accessibility. - Use CSS for styling instead of inline styles for better maintainability.
Exam Note: HTML Table
<!DOCTYPE html>
<html>
<head>
<title>Exam Note: HTML Table</title>
</head>
<body>
<table border="1">
<caption>Example Table</caption>
<tr>
<th>Header 1</th>
<th>Header 2</th>
</tr>
<tr>
<td>Data 1</td>
<td>Data 2</td>
</tr>
</table>
</body>
</html>
Header 1 | Header 2 |
---|---|
Data 1 | Data 2 |
Summary:
Today, we learned how to create and format tables in HTML. We covered the basic structure, adding headers and captions, spanning rows and columns, and styling tables using CSS. Remember to use best practices for better readability and accessibility.