Definition: Shell scripting in Linux involves writing a series of commands for the shell (a command-line interpreter) to execute. It’s like writing a program in which you specify a list of tasks that the computer should perform automatically.
Example of a Simple Shell Script
Here’s a basic example of a shell script:
echo "Hello, World!"
This script, when executed, prints “Hello, World!” to the screen.
Explanation in Points
- What is a Shell?
- The shell is a program that takes commands from the keyboard and passes them to the operating system to execute.
- Common shells in Linux include Bash (Bourne Again SHell), Zsh (Z Shell), and Fish (Friendly Interactive SHell).
- What is a Shell Script?
- A shell script is a file containing a series of commands.
- These commands are written in the shell’s scripting language.
- Purpose of Shell Scripting:
- Automate repetitive tasks.
- Simplify complex sequences of commands.
- Manage system operations and files.
- Schedule backups and monitor systems.
- How to Write a Shell Script:
- Use a text editor to write the script.
- Save the file with a
.sh
extension (e.g.,myscript.sh
).
- Basic Components of a Shell Script:
- Shebang (
#!/bin/bash
): The first line that tells the system which interpreter to use. - Commands: The tasks you want the script to perform, written line by line.
- Comments: Lines that begin with
#
are comments and are not executed.
- Shebang (
- How to Run a Shell Script:
- Make the script executable:
chmod +x myscript.sh
- Execute the script:
./myscript.sh
- Make the script executable:
- Benefits of Shell Scripting:
- Efficiency: Save time by automating repetitive tasks.
- Consistency: Ensure tasks are performed the same way every time.
- Control: Manage system processes and files easily.
- Common Commands Used in Shell Scripts:
echo
: Print text to the screen.ls
: List directory contents.cd
: Change directories.cp
,mv
,rm
: Copy, move, and remove files.if
,for
,while
: Control flow statements for conditional operations and loops.
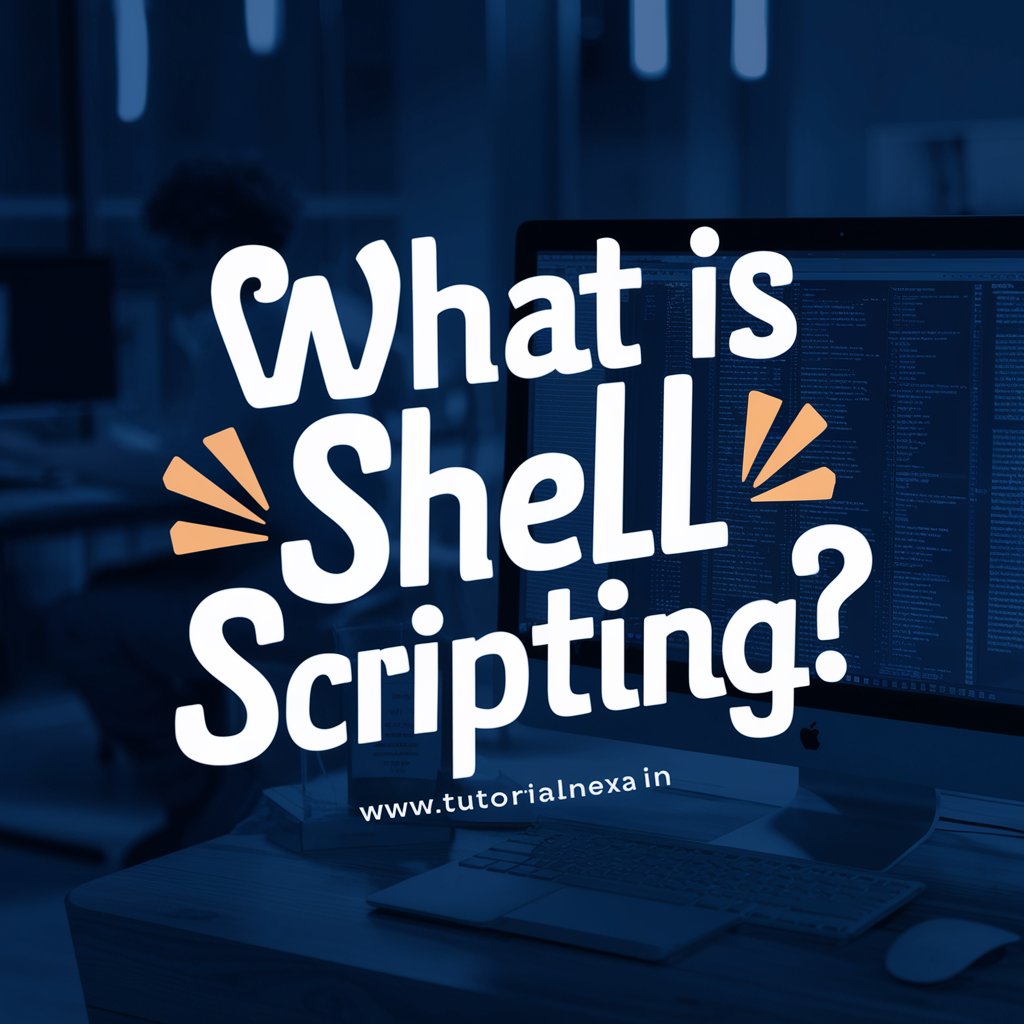
Important Points for Examination
- Definition: Shell scripting is writing a series of commands for the shell to execute automatically.
- Purpose: Automate tasks, manage systems, perform backups, and simplify complex operations.
- Shebang: The first line (
#!/bin/bash
) specifies the interpreter. - Executable Scripts: Use
chmod +x filename.sh
to make the script executable. - Common Commands: Learn basic commands like
echo
,ls
,cd
, and control flow statements (if
,for
,while
). - Advantages: Efficiency, consistency, and control over system operations.
By understanding these key points, you’ll be well-prepared to answer questions about shell scripting in a Linux environment during exams.
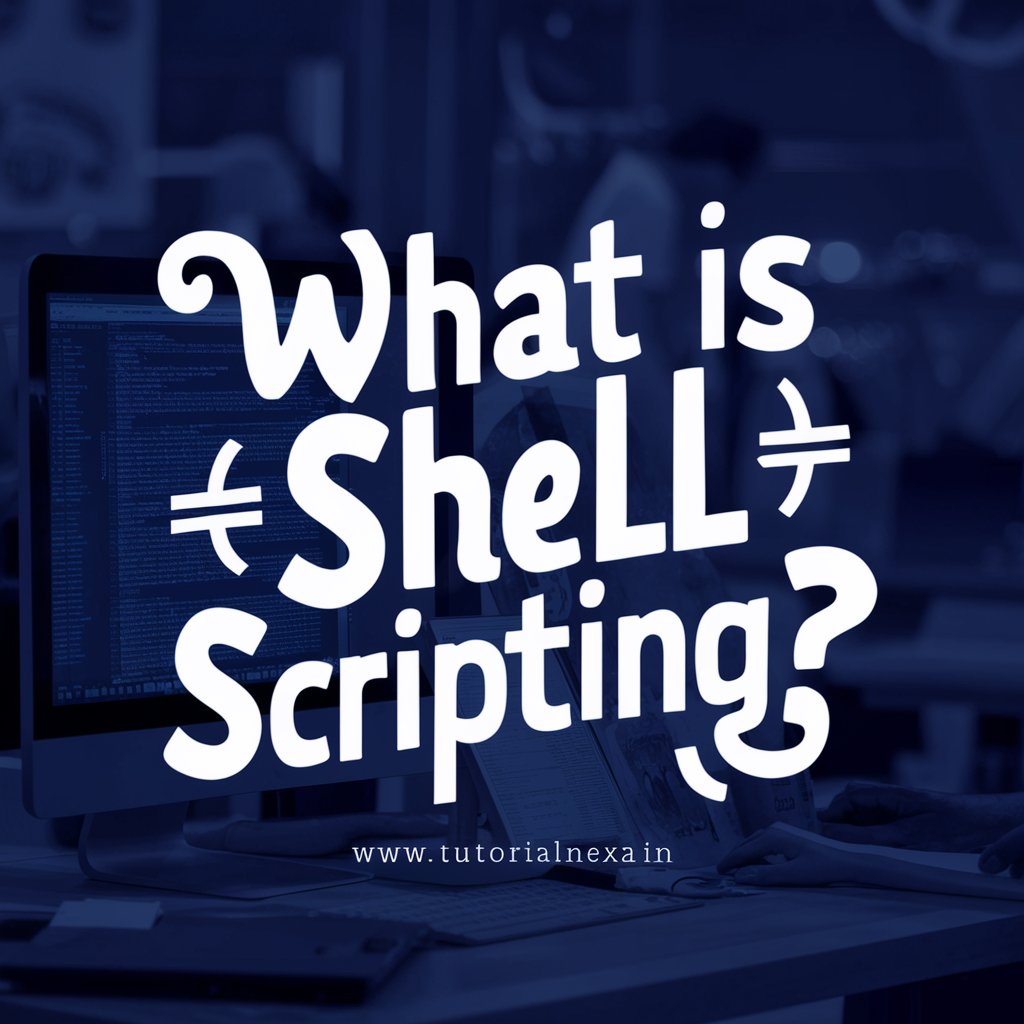
Detailed Information about Shell Scripting in Linux
Shell scripting is a powerful tool for automating tasks, managing systems, and simplifying complex operations in Linux. Here’s a more detailed overview:
Definition
Shell scripting is the process of writing a series of commands for a shell (command-line interpreter) to execute. These scripts are typically used to automate repetitive tasks, perform system administration, and facilitate complex processes.
Components of a Shell Script
- Shebang (
#!/bin/bash
):- The first line of a shell script, called the shebang, specifies the script’s interpreter.
- For example,
#!/bin/bash
tells the system to use the Bash shell to interpret the script.
- Commands:
- Shell scripts consist of standard Linux commands executed in sequence.
- Example commands:
ls
,cd
,mkdir
,cp
,mv
,rm
.
- Variables:
- Variables store data that can be used and manipulated throughout the script.
- Example:
my_var="Hello, World!"
.
- Control Structures:
- Conditional Statements:
if
,elif
,else
to perform actions based on conditions. - Loops:
for
,while
,until
to repeat actions multiple times. - Case Statements:
case
to handle multiple conditions.
- Conditional Statements:
- Functions:
- Functions allow you to group commands into reusable units.
- Example:
- my_function() {
- echo “This is a function”
- }
Steps to Create and Run a Shell Script
- Create the Script:
- Use a text editor (e.g.,
nano
,vim
,gedit
) to write your script. - Save the file with a
.sh
extension (e.g.,myscript.sh
).
- Use a text editor (e.g.,
- Make the Script Executable:
- Use the
chmod
command to make the script executable:chmod +x myscript.sh
.
- Use the
- Run the Script:
- Execute the script from the terminal:
./myscript.sh
.
- Execute the script from the terminal:
Key Concepts and Commands
- File Manipulation:
cp
: Copy files or directories.mv
: Move or rename files or directories.rm
: Remove files or directories.touch
: Create empty files or update file timestamps.
- Directory Operations:
mkdir
: Create directories.rmdir
: Remove empty directories.cd
: Change the current directory.pwd
: Print the current working directory.
- Text Processing:
echo
: Print text to the screen.cat
: Concatenate and display file content.grep
: Search text using patterns.awk
: Pattern scanning and processing language.sed
: Stream editor for filtering and transforming text.
- System Information:
uname
: Display system information.df
: Report filesystem disk space usage.top
: Display tasks and system performance.
- Networking:
ping
: Check network connectivity.curl
: Transfer data from or to a server.ssh
: Securely connect to remote servers.
Advanced Topics
- Script Debugging:
- Use
set -x
to enable debug mode. - Use
set +x
to disable debug mode.
- Use
- Error Handling:
- Use
trap
to catch signals and handle errors gracefully. - Example:
- Use
trap ‘echo “An error occurred. Exiting…”; exit 1;’ ERR
- Environment Variables:
- Use environment variables to store system-wide values accessible to all scripts and processes.
- Example:
export PATH=$PATH:/new/path
- Scheduling Scripts:
- Use
cron
to schedule scripts to run at specific times or intervals. - Example:
crontab -e
to edit cron jobs.
- Use
Summary
- Definition: Writing a series of commands for the shell to execute.
- Purpose: Automate tasks, manage systems, and simplify operations.
- Components: Shebang, commands, variables, control structures, functions, comments.
- Steps: Create, make executable, and run the script.
- Key Commands: File manipulation, directory operations, text processing, system information, networking.
- Advanced Topics: Script debugging, error handling, environment variables, scheduling scripts.
Understanding these concepts will help you effectively create and manage shell scripts in Linux, making your system administration tasks more efficient and less error-prone.